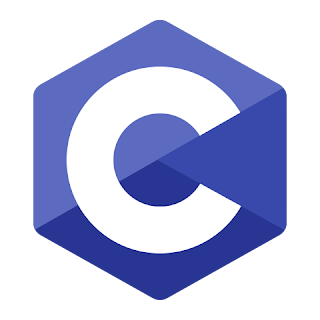
C Examples
C is a general-purpose programming language that has played a pivotal role in shaping modern software development. Known for its efficiency and close-to-hardware capabilities. C programming is an excellent language to learn to program for beginners.
Writing programs in C is the best way to learn C programming. The page contains examples programs for basic C concepts. You should go through all of these examples and attempt them on your own.
This page contains a list of c programs on Fundamental C concepts, Array, Strings, Matrix, Recursion, Geometrical and Mathematical problems. All of the example programs here are compiled successfully, executes and verified with sample input and output. Most of the program here will work on most standard compilers.
Feel free to Contact Us, If you find any bug in any of the below mentioned programs.