Here is C program to add two matrices of same dimensions. A matrix is a two-dimentional array of elements. The horizontal and vertical lines of elements in a matrix are called rows and columns of a matrix.
The size of a matrix is defined as the number of rows and columns of that matrix. A matrix with M rows and N columns is called a M × N matrix. The element in the i-th row and j-th column of a matrix A is referred as (i,j), or A[i,j]. We can perform a number of operations on matrices like addition, subtraction , multiplication, inverse, row and column operations etc.
- Matrix addition is defined for two matrices of the same dimensions, If the size of matrices are not same, then the sum of these two matrices is said to be undefined.
- The sum of two M × N matrices A and B, denoted by A + B, is again a M × N matrix computed by adding corresponding elements.
Let A and B are two matrices of dimension M X N and S is the sum matrix(S = A + B). Below mwntionws steps explains how we can find fum of two matrices.
- To add two matrices we have to add their corresponding elements. For example, S[i][j] = A[i][j] + B[i][j].
- Traverse both matrices row wise(first all elements of a row, then jump to next row) using two loops(check line number 30 and 31 of below program).
- For every element A[i][j], add it with corresponding element B[i][j] and store the result in Sum matrix at S[i][j].
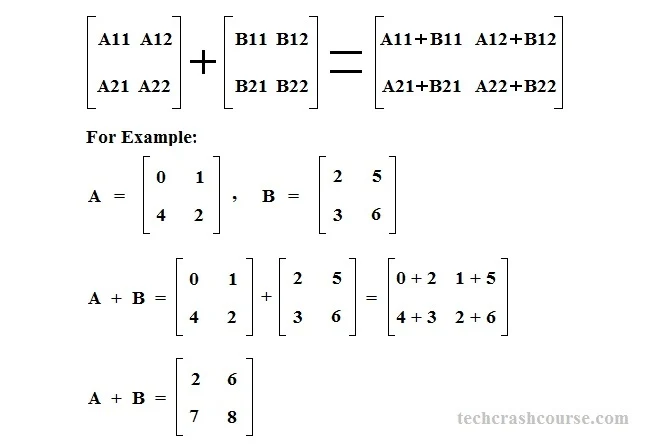
Properties of matrix addition
Let A, B, and C be M X N matrices, and let 0 denote the M X N zero matrix.- Associativity of Addition : (A + B) + c = A + (B + C)
- Commutativity of Addition : A + B = B + A
- Identity for Addition : 0 + A = A and A + 0 = A
C Program to add two matrix
#include <stdio.h> int main(){ int rows, cols, rowCounter, colCounter; int firstmatrix[50][50], secondMatrix[50][50], sumMatrix[50][50]; printf("Enter Rows and Columns of Matrix\n"); scanf("%d %d", &rows, &cols); printf("Enter first Matrix of size %dX%d\n", rows, cols); /* Input first matrix*/ for(rowCounter = 0; rowCounter < rows; rowCounter++){ for(colCounter = 0; colCounter < cols; colCounter++){ scanf("%d", &firstmatrix[rowCounter][colCounter]); } } /* Input second matrix*/ printf("Enter second Matrix of size %dX%d\n", rows, cols); for(rowCounter = 0; rowCounter < rows; rowCounter++){ for(colCounter = 0; colCounter < cols; colCounter++){ scanf("%d", &secondMatrix[rowCounter][colCounter]); } } /* adding corresponding elements of both matrices sumMatrix[i][j] = firstmatrix[i][j] + secondMatrix[i][j] */ for(rowCounter = 0; rowCounter < rows; rowCounter++){ for(colCounter = 0; colCounter < cols; colCounter++){ sumMatrix[rowCounter][colCounter] = firstmatrix[rowCounter][colCounter] + secondMatrix[rowCounter][colCounter]; } } printf("Sum Matrix\n"); for(rowCounter = 0; rowCounter < rows; rowCounter++){ for(colCounter = 0; colCounter < cols; colCounter++){ printf("%d ", sumMatrix[rowCounter][colCounter]); } printf("\n"); } return 0; }Output
Enter Rows and Columns of Matrix 2 2 Enter first Matrix of size 2X2 0 1 2 3 Enter second Matrix of size 2X2 1 2 2 3 Sum Matrix 1 3 4 6
Enter Rows and Columns of Matrix 2 3 Enter first Matrix of size 2X2 1 0 3 2 6 3 Enter second Matrix of size 2X2 5 1 1 2 0 1 Sum Matrix 6 1 4 4 6 4
Related Topics