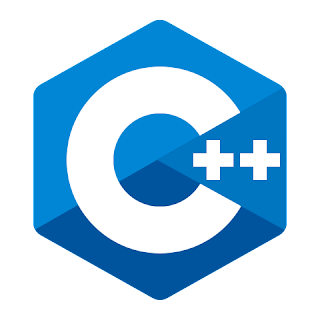
C++ Examples
C++ is a powerful and versatile programming language that combines the features of both high-level and low-level programming. Initially developed as an extension of the C programming language, C++ introduces object-oriented programming concepts.
C++ remains a popular choice for programmers seeking a balance between control and productivity in software development. Writing programs in C++ is the best way to learn C++ programming. The page contains examples programs for basic C++ concepts. You should go through all of these examples and attempt them on your own.
C++ Fundamental Programs
C++ Program to Print Hello World
C++ Program for Input and Output of Integer
C++ Taking Input From User
C++ Program to Perform Addition Subtraction Multiplication Division
C++ Program to Check Whether a Character is Alphabet or Not
C++ Program to Check Whether a Character is Vowel or Not
C++ Program to Count Zeros, Positive and Negative Numbers
C++ Program to Remove all Non Alphabet Characters from a String
C++ Program to Multiply two Numbers
C++ Program to Find Sum of Natural Numbers
C++ Program to Calculate Average Percentage Marks
C++ Program to Print a Sentence on Screen
C++ Program to Add Two Numbers
C++ Program to Read an Integer and Character and Print on Screen
C++ Program to Find Quotient and Remainder
C++ Program to Calculate Standard Deviation
C++ Program to Find Average of Numbers Using Arrays
C++ Program to Find Size of Variables using sizeof Operator
C++ Program to Swap Two Numbers
C++ Program to Swap Numbers in Cyclic Order
C++ Program to Check Whether Number is Even or Odd
C++ Program to Find LCM and GCD of Two Numbers
C++ Program to Check Whether a Character is Vowel or Consonant
C++ Program to Find Largest of Three Numbers
C++ Program to Find All Square Roots of a Quadratic Equation
C++ Program to Check Leap Year
C++ Program to Make a Simple Calculator
C++ Program to Generate Random Numbers
C++ Program to Find Factorial of a Number
C++ program to Find Factorial of a Number Using Recursion
C++ Program to Check Strings are Anagram or Not
C++ Program Linear Search in Array
C++ Program to Print Multiplication Table of a Number
C++ Program to Display Fibonacci Series using Loop and Recursion
C++ Program to Find GCD of Two Numbers
C++ Program to Reverse Digits of a Number
C++ Program to Convert Temperature from Celsius to Fahrenheit
C++ Program to Convert Fahrenheit to Celsius
C++ Program to Convert Decimal Number to Binary Number
C++ Program to Convert Decimal Number to Octal Number
C++ Program to Convert Decimal Number to HexaDecimal Number
C++ Program to Find Power of a Number
C++ Program to print ASCII Value of All Alphabets
C++ Program to Find Product of Two Numbers
C++ Program to check Whether a Number is Palindrome or Not
C++ Program to Check Prime Number
C++ Program to Print All Prime Numbers Between 1 to N
C++ Program to Check for Armstrong Number
C++ Program to Display Armstrong Number Between Two Intervals
C++ Program to Display Factors of a Number
C++ Program to Check Prime Number Using Function
C++ program to Check Whether a Number can be Split into Sum of Two Prime Numbers
C++ Program to Find Sum of Natural Numbers Using Recursion
C++ Program to Find GCD or HCF of Two Numbers Using Recursion
C++ Program to Find Power of Number using Recursion
C++ Program to Calculate Grade of Student Using Switch Case
C++ Program to Find Largest Element of an Array
C++ Program to Find Smallest Element in Array
C++ Program to Print Array in Reverse Order
C++ Program to Delete Spaces from String or Sentence
C++ Program to Delete Vowels Characters from String
C++ Program to Count Words in Sentence
C++ Program to Delete a Word from Sentence
C++ Program to Convert Uppercase to Lowercase Characters
C++ Program to Find Transpose of a Matrix
C++ Program to Add Two Matrix
C++ Program to Multiply Two Matrices
C++ Program to Access Elements of an Array Using Pointer
C++ Program to Find the Frequency of Characters in a String
C++ Program to Count Number of Vowels, Consonant, and Spaces in String
C++ Program to Check whether a string is Palindrome or Not
C++ Program to find length of string
C++ Program to concatenate two strings without using strcat function.
C++ Program to Copy String Without Using strcpy
C++ Program to Multiply Two Numbers Using Addition
C++ Program to Store Information of a Book in a Structure
C++ Program to Calculate Difference Between Two Time Periods
C++ Program to Add Two Complex Numbers Using Structure
C++ Program to Add Two Distances in Inch and Feet
C++ Program to Store Information of an Employee in Structure
C++ Program to Find Area and Circumference of a Circle
C++ Program to Find Area and Perimeter of Parallelogram
C++ Program to Print Floyd Triangle
C++ Program to Print Pascal Triangle