Here is a C program to find the area and perimeter of a rhombus. A rhombus is quadrilaterals(having four sides) with all sides equal in length and opposites sides parallel. Opposite angles of a rhombus are also equal. A rhombus is a special case of parallelogram where all sides are equal in length.
- Diagonals of a rhombus are perpendicular and divides each other in two equal half.
- Opposite sides of a rhombus are parallel and all sides are equal in length.
- Opposite angles of a rhombus are equal.
- The sum of any two adjacent angles of a rhombus are 180 degrees.
- The diagonal of rhombus bisects the interior angle.
To calculate the area of rhombus we need length of Base and Height of length of both diagonals.
Base : We can choose any side of a rhombus as base to calculate area of rhombus.
Height : Height of a rhombus is the perpendicular distance between the base and it's opposite side.
Diagonals : A line segment joining opposite vertices of a rhombus.
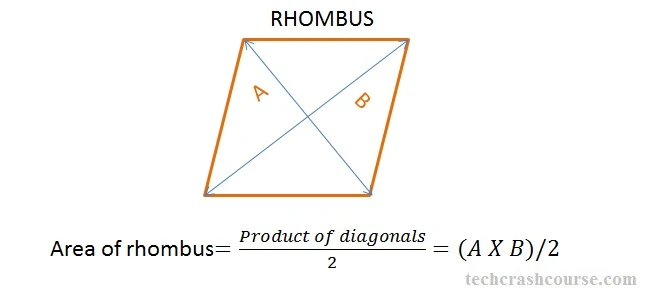
- Area of Rhombus = B X H
H is the length of height of Rhombus.
(Base and Height are perpendicular on each other)
If we know the length of both diagonals of a rhombus the, it's area can be calculated by multiplying length of both diagonals and then dividing it by 2.
- Area of Rhombus = (Product of diagonals)/2 = (A X B)/2
C Program to find the area of the rhombus
To calculate the area of a rhombus we need the length of both diagonals of a rhombus. Below program first takes the length of diagonals as input from user and stores it in two floating point numbers. Now, to find the area of rhombus we take semi product of diagonals. It finally prints the area of rhombus on screen using printf function.
#include <stdio.h> int main(){ float diagonalOne, diagonalTwo, area; printf("Enter the length of diagonals of rhombus\n"); scanf("%f %f", &diagonalOne, &diagonalTwo); area = (diagonalOne * diagonalTwo)/2; printf("Area of rhombus : %0.4f\n", area); return 0; }Output
Enter the length of diagonals of rhombus 3.5 4 Area of rhombus : 7.0000
C Program to find the perimeter of rhombus
The perimeter of a rhombus is the linear distance around the boundary of the rhombus. In other words, we can think of perimeter of a rhombus as the length of fence needed to enclose a rhombus.
- Perimeter of Rhombus = 4 X S
#include <stdio.h> int main(){ float side, perimeter; printf("Enter the length of side of rhombus\n"); scanf("%f", &side); perimeter = 4 * side; printf("Perimeter of rhombus : %0.4f\n", perimeter); return 0; }Output
Enter the length of side of rhombus 6.5 Perimeter of rhombus : 26.0000
To calculate the perimeter of rhombus, we need length of side of rhombus. Above program, first takes length of any side of rhombus as input from user and stores it in a floating-point variable. Then, we multiply length of side by 4 and store the result in a floating point variable called 'perimeter'. Finally, it prints the perimeter of Rhombus on screen using printf function.
- A rhombus has rotational symmetry.
- The shape of basketball ground is rhombus.
- A square is also a rhombus, having equal sides and all interior angles as 90 degrees.
- A rectangle is also a rhombus, having opposites sides equal parallel and all interior angles as 90 degrees.
- A rhombus is a special case of parallelogram, where all sides are equal.
- The diagonals of rhombus intersect at right angle.