Here is a C program to print rectangular star (*) pattern of n rows and m columns using for loop. For a rectangular star pattern of 5 rows and 9 columns. Program's output should be:
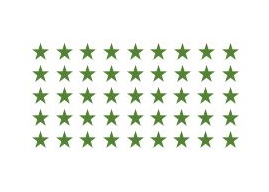
Printing rectangular star patterns is a fundamental programming exercise that helps beginners understand the concepts of loops, conditional statements, and basic arithmetic operations in C. In this article, we'll explore the advantages of this pattern printing program, its importance for beginners, and some tips for writing it effectively.
Required Knowledge
- Take the number of rows(N) and columns(M) of rectangle as input from user using scanf function.
- We will use two for loops to print rectangular star pattern.
- Outer for loop will iterate N times. In each iteration, it will print one row of pattern.
- Inner for loop will iterate M times.In one iteration, it will print one star (*) characters in a row.
Here is the matrix representation of the rectangle star pattern. The row numbers are represented by i whereas column numbers are represented by j.
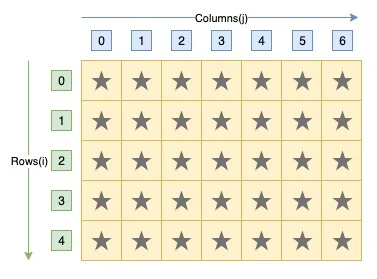
C program to print rectangular star pattern
#include<stdio.h> int main(){ int rows, cols , i, j; printf("Enter rows and columns of rectangle\n"); scanf("%d %d", &rows, &cols); /* Row iterator for loop */ for(i = 0; i < rows; i++){ /* Column iterator for loop */ for(j = 0; j < cols; j++){ printf("*"); } printf("\n"); } return 0; }Output
Enter rows and columns of rectangle 3 9 ********* ********* *********
Importance of Practicing Rectangular Star Pattern Printing Programs for Beginners
- Comprehending the concepts of looping and conditional statements : The program for printing a rectangular star pattern aids novice learners in comprehending the notion of nested loops. Beginners acquire the ability to regulate the sequence of actions in a program by utilizing nested loops to display rows and columns of asterisks, as well as employing conditional statements.
- Developing Self-Assurance : Mastering the skill of designing and executing a program that prints a rectangular star pattern can greatly enhance the confidence of a novice. It showcases their proficiency in implementing programming principles in a real-world context and inspires them to take on more intricate programming tasks.
- Acquiring Basic Programming Principles : The program for producing a rectangular star pattern instructs novice programmers on essential programming principles, including loops, conditional statements, and variable manipulation. These ideas are fundamental to programming and are crucial for novices to understand.
Tips for Writing Rectangular Star Pattern Printing Programs in C
- Employ nested loops : To generate a rectangular star pattern, utilize nested loops, with one loop for rows and another for columns. The outer loop governs the quantity of rows, whereas the inner loop governs the quantity of stars in each row.
- Utilize variables to represent size : Utilize variables to store the dimensions of the rectangle in order to enhance the program's adaptability. This feature enables effortless adjustment of the rectangle's dimensions without the need to make any alterations to the code.
- Conduct a step-by-step evaluation : Commence by creating a diminutive rectangle and progressively augment its dimensions in order to evaluate the functionality of your software. By identifying and rectifying faults at an early stage, you may guarantee the correct functionality of your application for rectangles of any dimension.
- Use comment blocks : Include explanatory comments in your code to elucidate the rationale behind each step. Using clear and concise code improves its comprehensibility for both others and oneself, which proves advantageous when reviewing the code at a later time.
Beginners can strengthen their comprehension of looping and conditional expressions, improve their problem-solving abilities, and establish a strong programming foundation by delving into the C program that prints a rectangular star pattern. Novice individuals can achieve mastery in the skill of pattern printing and discover boundless opportunities for innovative patterns through consistent practice and perseverance.
Related Topics