Here is a C program to print palindrome triangle pattern of n rows. This C Program to Print Palindrome Triangle Pattern is an intriguing exercise that combines the concepts of palindromes and patterns in programming. Exploring the Palindrome Triangle Pattern Program in C offers you a unique opportunity to enhance your understanding of palindromes, pattern recognition, nested loops, algorithmic thinking, and string manipulation concepts. It equips you with valuable skills that are essential for your growth as a programmer.
Palindrome triangle pattern of 5 rows:
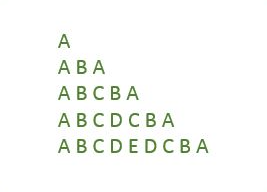
Required Knowledge
This program is silimar to right triangle star pattern program.
- Take the number of rows(N) of right triangle as input from user using scanf function.
- Number of characters in Kth row is always 2K+1. 0th row contains 1 character, 1st row contains 3 characters, 2nd row contains 5 charcaters and so on.
- In any row, we will first print the characters in increasing order of their ASCII value till mid of the row and then in decreasing order.
- We will use two for loops to print right triangle of palindrome strings.
- Outer for loop will iterate N time(from i = 0 to 2N-2 in increment of 2). Each iteration of outer loop will print one row of the pattern.
- Each iteration of inner loop will print a palindrome string as explained above.
Here is the matrix representation of the above mentioned pattern. The row numbers are represented by i whereas column numbers are represented by j.
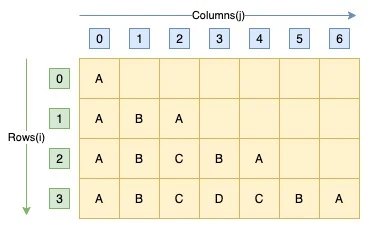
C program to print palindrome triangle pattern
#include<stdio.h> int main() { int i, j, rows, count=0; printf("Enter the number of rows\n"); scanf("%d", &rows); for (i = 0; i < 2*rows; i=i+2) { for (j = 0; j <= i; j++) { printf("%c", 'A'+count); if(j < i/2) count++; else count--; } count = 0; printf("\n"); } return(0); }Output
Enter the number of rows 5 A ABA ABCBA ABCDCBA ABCDEDCBA
Advantages of the Palindrome Triangle Pattern Program in C
- Understanding Palindromes : This program provides a practical application for understanding palindromes. It demonstrates how palindromes, which are sequences that read the same forwards and backwards, can be used in a pattern. This helps you grasp the concept of palindromes more effectively.
- Enhancement of Pattern Recognition Skills : Designing and implementing the Palindrome Triangle Pattern Program challenges you to recognize and understand palindrome patterns. It improves your ability to identify and analyze patterns in a sequence of characters, a skill that is valuable in programming.
- Improvement of Algorithmic Thinking : The program encourages you to think algorithmically, devising a strategy to generate and display palindrome patterns. This improves your ability to break down a complex problem into logical steps, a crucial skill in programming.
- Introduction to Nested Loops : Writing this program requires the use of nested loops to control the number of rows and columns printed in each iteration. Nested loops are a fundamental concept in programming, and this program introduces you to their use in a practical context.
- Application of String Manipulation Concepts : Implementing this program enhances your understanding of string manipulation concepts, such as reversing a string to form a palindrome. It provides you with hands-on experience in applying these concepts, which are essential in many programming tasks.
- Preparation for Advanced Programming Challenges : The skills and concepts you learn from this program serve as a foundation for tackling more advanced programming challenges. It prepares you for problems that require algorithmic thinking, pattern recognition, and efficient use of loops.
Related Topics