Here is a C program to print hollow rectangular star pattern of n rows and m columns. For a hollow rectangular star pattern of 4 rows and 12 columns. Program's output should be:
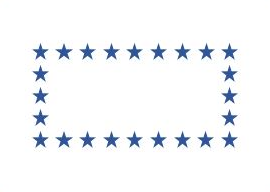
Required Knowledge
The algorithm of this pattern is similar to rectangular star pattern except here we have to print stars of first and last rows and columns only. At non-boundary position, we will only print a space character.
- Take the number of rows(N) and columns(M) of rectangle as input from user using scanf function.
- We will use two for loops to print empty rectangular star pattern.
- Outer for loop will iterate N times. In each iteration, it will print one row of pattern.
- Inside inner for loop, we will add a if statement check to find the boundary positions of the pattern and print star (*) accordingly.
Here is the matrix representation of the hollow rectangle star pattern. The row numbers are represented by i whereas column numbers are represented by j.
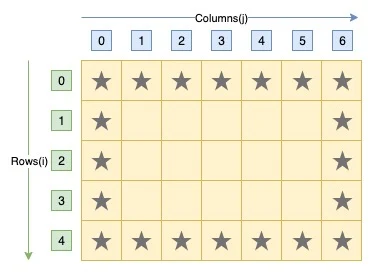
C program to print hollow rectangular star pattern
#include<stdio.h> int main(){ int rows, cols , i, j; printf("Enter rows and columns of rectangle\n"); scanf("%d %d", &rows, &cols); /* Row iterator for loop */ for(i = 0; i < rows; i++){ /* Column iterator for loop */ for(j = 0; j < cols; j++){ /* Check if currely position is a boundary position */ if(i==0 || i==rows-1 || j==0 || j==cols-1) printf("*"); else printf(" "); } printf("\n"); } return 0; }Output
Enter rows and columns of rectangle 4 12 ************ * * * * ************
Tips for Writing the Hollow Rectangle Star Pattern Printing Program in C
- Variable Use for Size Flexibility : Use variables to store the width and height of the rectangle, enabling easy customization and modification of the program.
- Conditional Statements for Shape Definition : Incorporate conditional statements to determine when to print stars and when to print spaces, ensuring the accurate depiction of the hollow rectangle shape.
- Incremental Testing : Begin with a small hollow rectangle and gradually increase its size to test the program, identifying and rectifying errors early in the development process.
- Utilize Nested Loops : Employ nested loops—one for rows and another for columns—to systematically print rows and columns of stars, forming the hollow rectangle shape.
Conclusion
Finally, the Hollow Rectangle Star Pattern Printing Program in C is a basic exercise that helps novices develop their problem-solving abilities, strengthen their understanding of looping, sharpen their debugging techniques, and foster their creativity in programming. For novices to understand basic programming principles and develop confidence in their programming skills, practicing this program is essential. Learners can put this curriculum into practice and lay a strong basis for their programming journey by following the advice given.
Related Topics