Decision making conditional statements allow a program to take a different path depending on some condition(s). Decision making statements(like if, if-else, if-else ladder, switch statement) lets us evaluate one or more conditions and then take decision whether to execute a block of code or not, or to execute which block of code.
It allow a java program to perform a test and then take action based on the result of that test. Using decision making statements, we can change the behaviour of our program based on certain condition. In other words, using decision making statements, we can specify different action items for different situations to make our application more agile and adaptive.
- If Statement
- If else statement
- If else Ladder
- Nested If Statement
- Switch case Statement
If Statement in Java
If statement in java first evaluates a conditional expression. If conditional expression evaluates to true then only block of code inside if gets executed otherwise control goes to the statement after if block.
If block is used to execute a set of statements only if some condition(s) becomes true.
if(condition_expression) { statements; }
condition_expression is a boolean expression (evaluates to true or false like i > 100)
- If condition_expression evaluates to true, statements are executed.
- If condition_expression evaluates to false, statements are skipped.
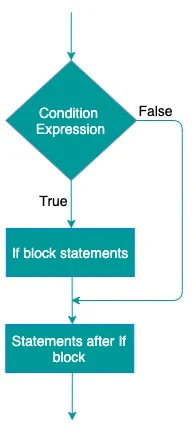
Java If Statement Example Program
public class IfStatement { public static void main(String[] args) { int num = 10; if (num > 0) { System.out.println("Positive Number"); } System.out.println(num); } }Output
Positive Number 10Following If statement checks if the value of num is greater than 0. If the condition is true, it executes the code inside the curly braces, which prints "Positive Number" to the console.
if (num > 0) { System.out.println("Positive Number"); }
Best Practices of Using If Statement
- Use Clear and Descriptive Conditions : When writing conditions for your if statements, make sure they are clear and easily understandable. Use meaningful variable names and avoid complex expressions that may be confusing. Additionally, use parentheses to explicitly specify the order of evaluation, especially when combining multiple conditions.
- Avoid Nested If Statements if Possible : While nested if statements can be necessary, excessive nesting can make your code harder to read and maintain. Consider refactoring nested if statements into separate methods or using alternative control structures like switch statements or polymorphism.
- Use Switch for Multiple Conditions : If you are dealing with a series of conditions based on the value of a single variable, consider using a switch statement. This can make your code more readable and maintainable, especially when dealing with a large number of cases.
- Keep Code DRY (Don't Repeat Yourself) : If you find yourself repeating similar conditions in multiple places, consider creating a method to encapsulate the logic. This promotes code reuse and makes it easier to maintain and update your application.
- Handle Edge Cases : Ensure that your if statements cover all possible scenarios, including edge cases and unexpected inputs. Consider using additional conditions or validation checks to handle special cases that may lead to unexpected behavior if not accounted for.
- Test Your Code : Thoroughly test your if statements with different inputs to verify that your logic works as intended. Test not only the common scenarios but also edge cases and boundary conditions. Automated testing frameworks can be particularly helpful in ensuring the reliability of your code.
Points to Remember about If Statement
- condition_expression must evaluate to true or false.
- We can combine multiple conditions to using logical operators(&& and ||) to form a compound conditional expression.
For Example:if(condition_one && condition_two) { /* if code block */ }
- Opening and Closing Braces are optional, If the block of code of if statement contains only one statement.
For Example:if(condition_expression) statement1; statement2;
In above example only statement1 will gets executed if condition_expression is true.
Conclusion
The if statement is a fundamental building block in Java programming, enabling you to make decisions and control the flow of your code. By understanding its syntax, various forms, and best practices, you can write more readable, maintainable, and bug-free code. Whether you are a beginner or an experienced developer, mastering the if statement is essential for writing effective Java programs. As you gain proficiency, you'll find yourself using if statements in conjunction with other control flow structures to create powerful and flexible applications.