
Design Patterns in Java
A design pattern is a reusable generic solution to a frequent problem in software design. Design patterns are recommended practices that experienced developers employ to overcome common challenges.
Knowing merely principles such as inheritance, polymorphism, and encapsulation will not qualify you as an excellent object oriented developer. You must consider developing reusable, adaptable, and simple-to-maintain systems. To handle certain common design difficulties, you need be familiar with at least a few design patterns.
In this tutorial, we will explore the basics of design patterns, their importance, and how they can be implemented in Java. We will cover three fundamental categories of design patterns: creational, structural, and behavioral.
The Gang of Four (GoF) - Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides - introduced 23 classic design patterns in their book "Design Patterns: Elements of Reusable Object-Oriented Software," which is considered a cornerstone in the field of software design.
Why Design Patterns are Recommended
Design patterns are general repeatable solutions to common problems in software design. They are templates that can be adapted to solve a variety of problems in different contexts. Design patterns can speed up the development process by providing tested, proven development paradigms.
Design patterns are essential tools in software development that provide reusable solutions to common problems. They represent best practices and encapsulate the knowledge and experience of seasoned developers. Using design patterns in your Java applications can lead to more maintainable and scalable code.
Types of Design Patterns
Design patterns can be broadly classified in three categories: Creational, Structural and Behavioral design patterns.
- Creational Patterns : These design patterns deal with the problem of creating object while hiding the logic and complexity associated with it. They help to make a system independent of its object creation, composition, and representation. Singleton Pattern, Factory Pattern, Builder Pattern etc comes under creational design patterns.
- Structural Patterns : These design patterns are used to define relationship between classes, objects and various entities. Structural patterns are concerned with the composition of classes or objects. They help to assemble groups of objects into larger structures, yet keeping these structures flexible and efficient. Adapter Pattern, Bridge Pattern, Proxy Pattern etc comes under structural design patterns.
- Behavioral Patterns : These patterns are concerned with the interaction and responsibility of objects.They focus on how objects communicate and collaborate with each other. Command Pattern, Mediator Pattern, Observer Pattern, Strategy Pattern etc comes under behavioral patterns.
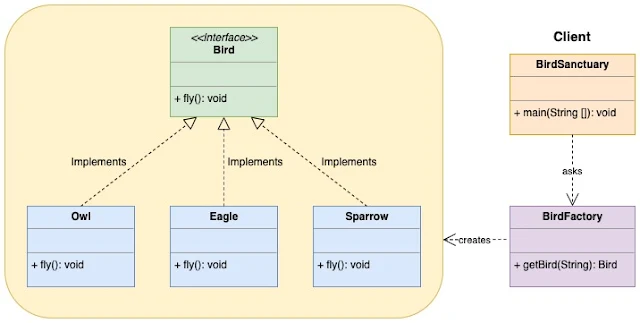
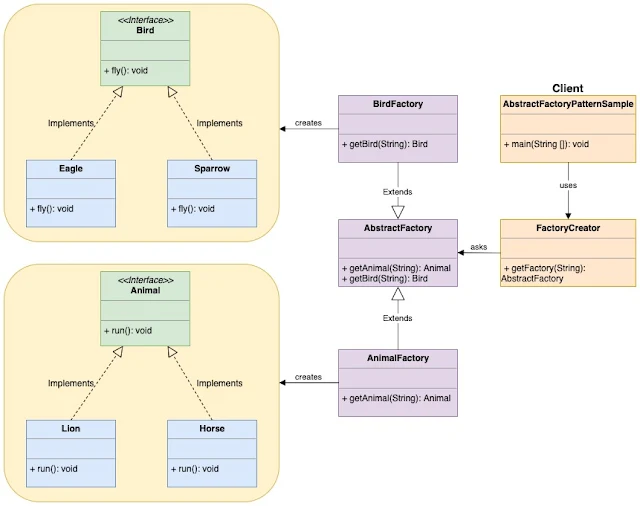
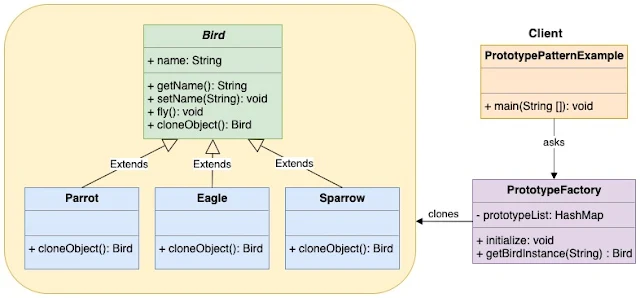
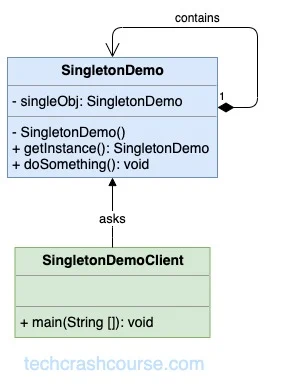

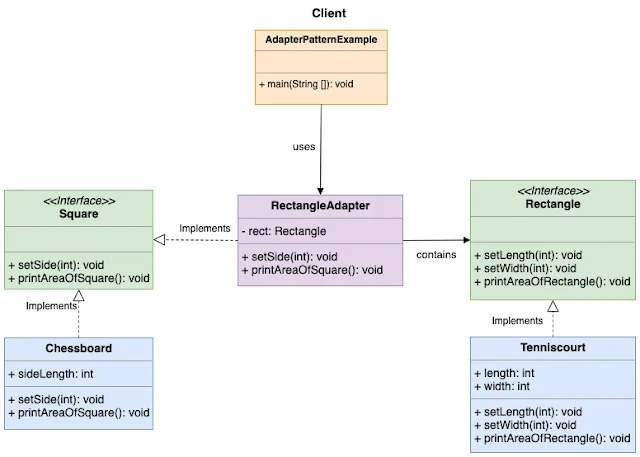
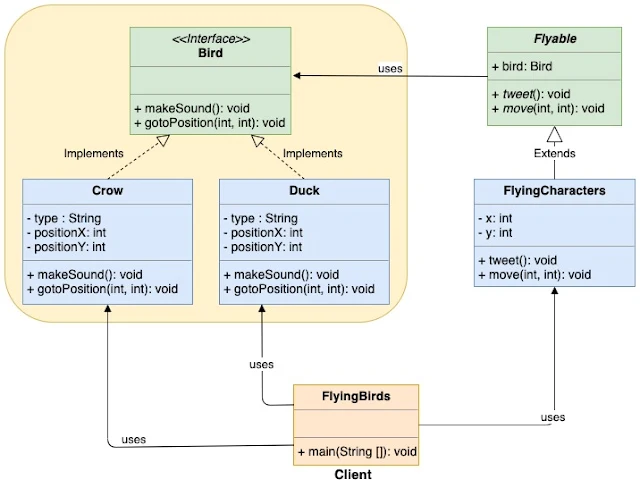
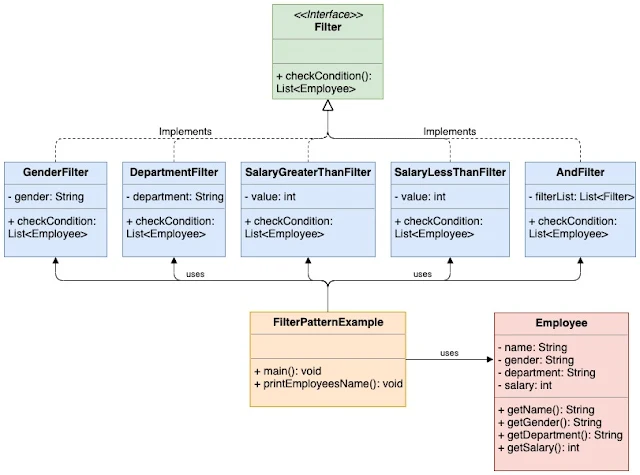
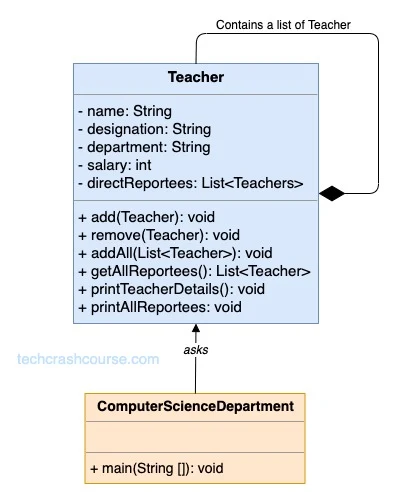
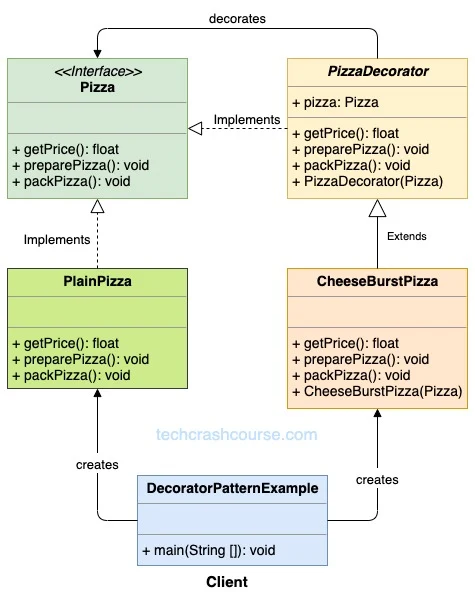
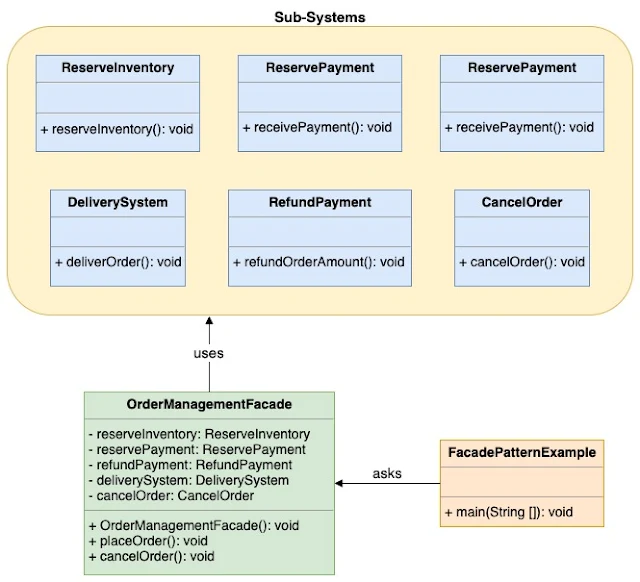
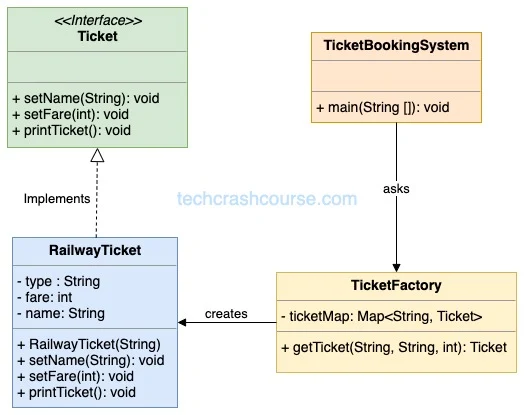
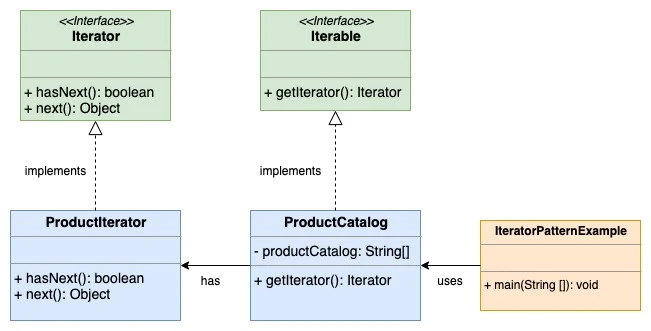
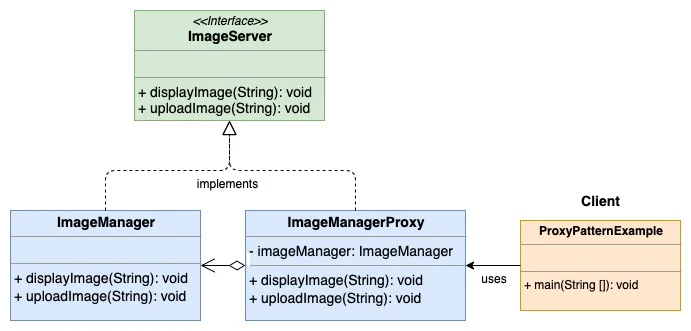
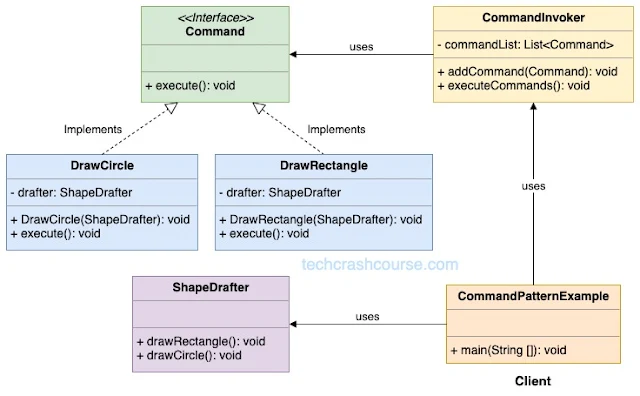
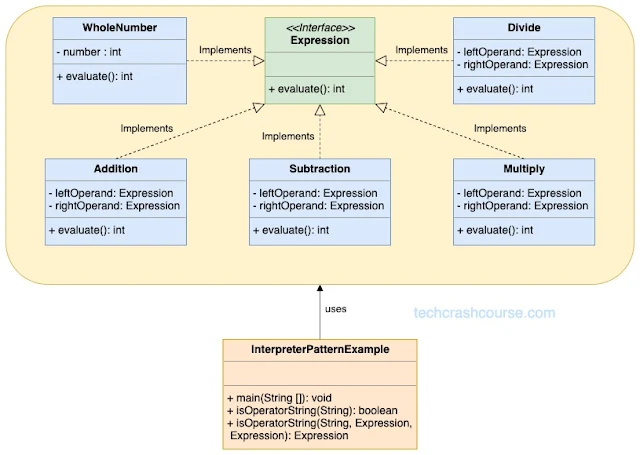
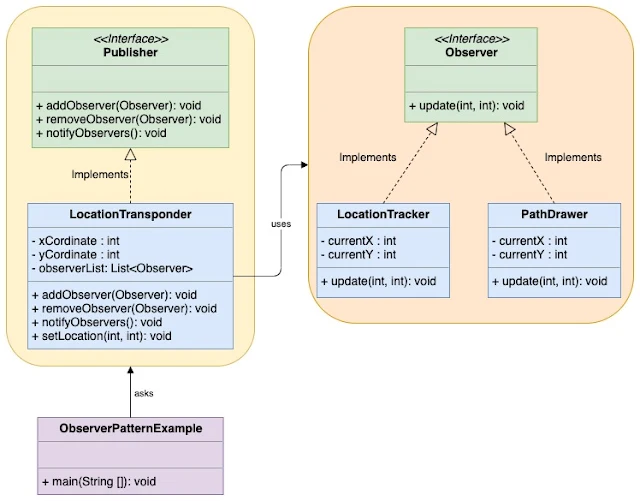
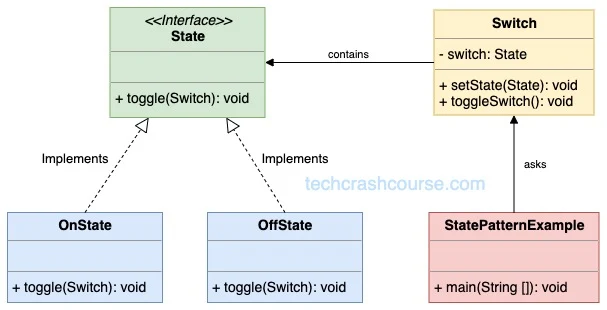
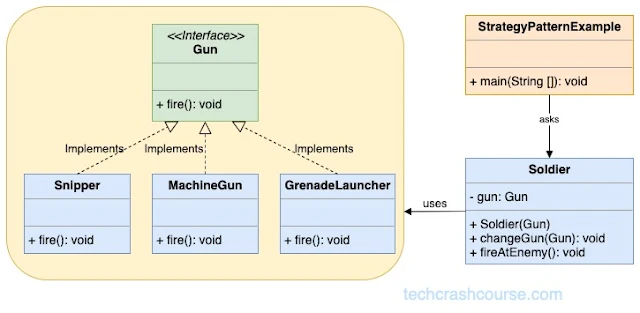
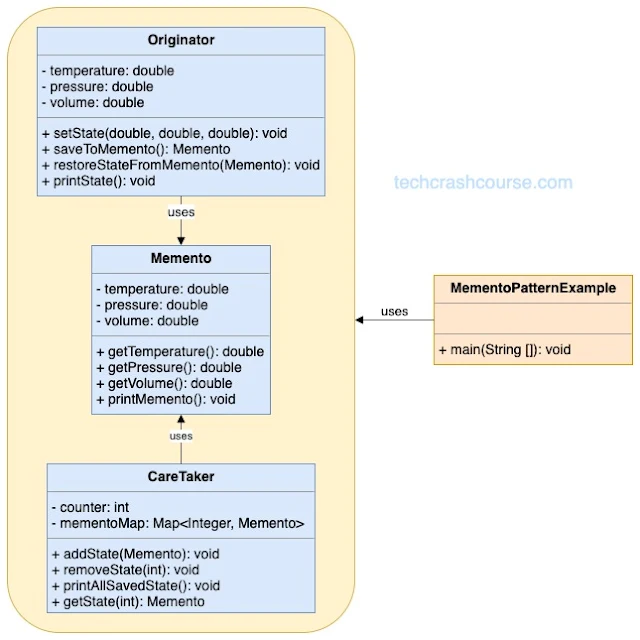
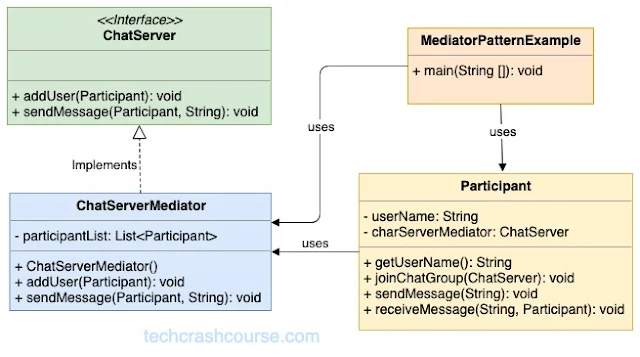
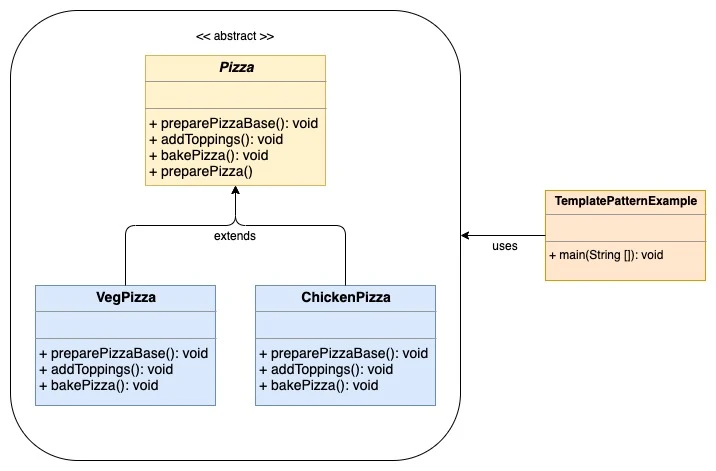
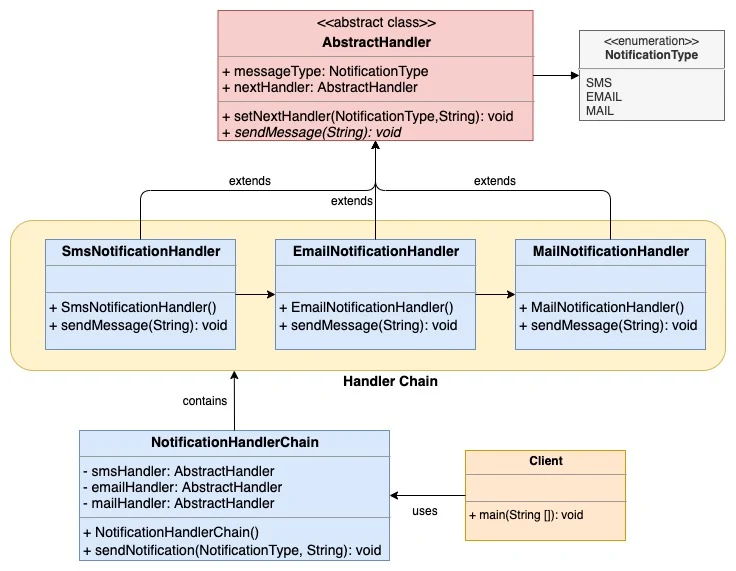
Principles of Design Patterns
- Identify the components of your system that varies and keep them separate from what remains the same. In this way, you can change or extend the components that vary independently without affecting the components that remains same.
- Program to an interface not an implementation.
- Favour composition over inheritance. In other words, a "HAS-A relationship is better than IS-A".
Advantages of Design Patterns
- Design Patterns helps you to build reusable systems with good object oriented design qualities.
- They capture the proven object oriented experiences. You don't have to re-invent again.
- It provides shared vocabularies that allows you to say more with less. It helps developers to communicate efficiently.
- Design patterns let you write better code more quickly.
- It specifies the best practices and solutions used the developers everywhere to solve a particular problems faced during software development.
- Open/Closed Principle (OCP) : This principle encourages developers to extend the behavior of a system without modifying its existing code. By using interfaces, abstract classes, and polymorphism, new functionalities can be added without altering the existing codebase.
- Single Responsibility Principle (SRP) : This principle promotes the idea that a class should encapsulate a single responsibility or functionality. This helps in making classes more maintainable and understandable. If a class has more than one reason to change, it's advisable to split it into multiple classes.
- Liskov Substitution Principle (LSP) : This principle ensures that derived classes can be used interchangeably with their base classes. It reinforces the idea that inheritance should not break the functionality of the base class. Violating the Liskov Substitution Principle can lead to unexpected behavior in the application.
- Dependency Inversion Principle (DIP) : This principle suggests that high-level modules (e.g., business logic) should not be dependent on low-level modules (e.g., specific implementations). Instead, both should depend on abstractions (interfaces or abstract classes). This promotes flexibility and decouples high-level modules from specific implementations.
- Interface Segregation Principle (ISP) : This principle advocates for the creation of specific interfaces for clients rather than a general-purpose one. Classes should not be burdened with implementing methods they do not need. By having smaller, focused interfaces, developers can avoid unnecessary dependencies.
- Composition Over Inheritance : While inheritance can be powerful, it can also lead to rigid class hierarchies and issues like the diamond problem. Composition promotes flexibility, reusability, and easier maintenance. By favoring composition, developers can build systems that are more modular and extensible.
- Encapsulate What Varies : By encapsulating the parts of the code that are subject to change, developers can isolate the impact of modifications and make the system more robust. This principle aligns with the Open/Closed Principle and encourages modular design.
- Favor Object Composition Over Class Inheritance : While inheritance can introduce complexity and coupling, object composition allows developers to assemble systems from smaller, independent components. This approach often leads to more maintainable and adaptable code.
Conclusion
Design patterns are of paramount importance in the realm of software development as they facilitate code reusability and offer remedies for recurring issues. We have examined the fundamentals of design patterns in this tutorial, with an emphasis on structural, behavioral, and creational patterns. The utilization of design patterns in Java applications can result in improvements in scalability, flexibility, and maintainability. As your understanding of software design progresses, contemplate the examination and application of supplementary design patterns in order to augment your programming proficiencies.