Loop control statements in C are used to execute a block of code several times until the given condition is true. Whenever we need to execute some statements multiple times, we need to use a loop statement.
Loops in C are used in performing repetitive tasks. Here are some situations where we should use loops:
- We want to find sum of all consecutive integers between 0 to N.
- You want to take N numbers as input from user and store it in an array. Where N is not fixed, it is based on user input.
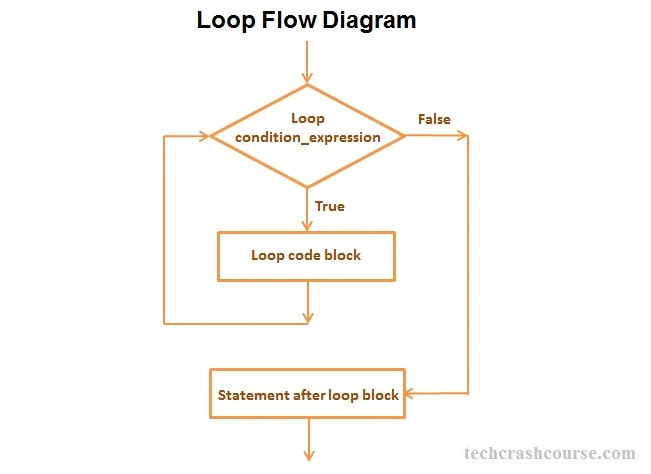
Types of Loop Statements in C
Click on the links below to check detailed description of various loop statements.Loop Type | Description |
---|---|
for loop | Repeats a block of code multiple times until a given condition is true. Initialization, looping condition and update expression(increment/decrement) is part of for loop syntax. |
while loop | Repeats a block of code multiple times until a given condition is true. Unlike for loop, while loop doesn't contain initialization and update expression in it's syntax. |
do while loop | Similar to while loop, but it tests the condition at the end of the loop body. The block of code inside do while loop will execute at least once. |
Break Statement in C
The break statement in C programming language is used to terminate loop and takes control out of the loop. We sometimes want to terminate the loop immediately without checking the condition expression of loop. The break statement is used with conditional if statement.
Use of break statement, change the normal sequence of execution of statements inside loop.
Syntax of break Statement
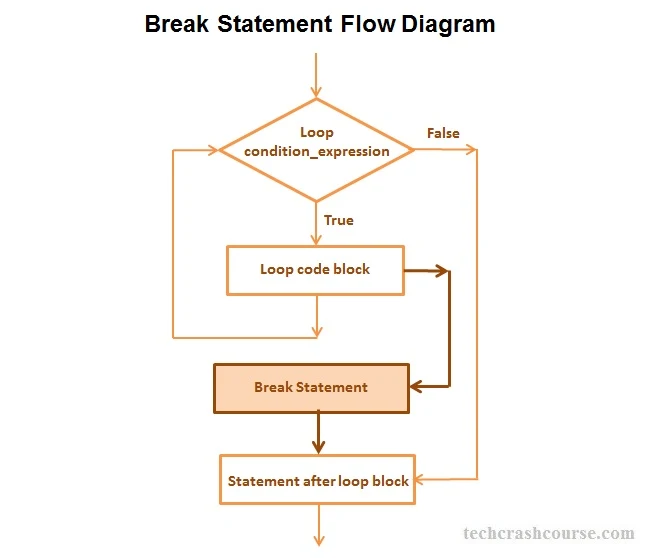
Use of break Statement
- We can use break statement inside any loop(for, while and do-while). It terminates the loop immediately and program control resumes at the next statement following the loop.
- We can use break statement to terminate a case in the switch statement.
C Program to show the use of break statement
#include <stdio.h> int main(){ /* * Using break statement to terminate for loop */ int N, counter, sum=0; printf("Enter a positive number\n"); scanf("%d", &N); for(counter=1; counter <= N; counter++){ sum+= counter; /* If sum is greater than 50 break statement will terminate the loop */ if(sum > 50){ printf("Sum is > 50,terminating loop\n"); break; } } if(counter > N) printf("Sum of Integers from 1 to %d = %d", N, sum); return(0); }Above program find sum of all integers from 1 to N, using for loop. It sum is greater than 50, break statement will terminate for loop.
Output
Enter a positive number 9 Sum of Integers from 1 to 9 = 45
Enter a positive number 10 Sum is > 50, terminating loop
Best Practices for Using the Break Statement in C
- Avoid Nested Loop Breaks :Refrain from utilizing the break statement as a means to terminate nested loops. Alternatively, contemplate utilizing a flag variable or other control techniques to terminate both loops together. Using the break statement to exit nested loops might result in code that is more difficult to comprehend and maintain.
- Use Break Sparingly in Switch Statements : Within a switch statement, the break statement is employed to terminate the switch block as soon as a matching case is encountered. Proper utilization of the break statement is crucial in order to prevent inadvertent execution of succeeding instances. It is generally recommended to conclude each instance with a break statement, unless there is a conscious intention to continue to the next case without a pause.
- Consider Using Return Instead of Break : When dealing with functions that have the main objective of executing a certain task and terminating prematurely depending on a condition, it is advisable to employ the return statement. By making the code more transparent, superfluous else blocks are avoided.
- Test Thoroughly : When using break statements, particularly in intricate control flow scenarios, ensure to properly test your code utilizing various inputs and edge cases. Verify that the control flow operates as intended and that there are no unwanted instances of falling through or exiting prematurely.
- Provide Clear Conditions : Ensure that the criteria that cause the break statement to be executed are clearly defined and thoroughly documented. Enhancing code readability facilitates comprehension of the reasoning by others, including oneself.
Continue Statement in C
The continue statement in C programming language is used to skip some statements inside the body of the loop and continues the next iteration of the loop. The continue statement only skips the statements after continue inside a loop code block.
The continue statement is used with conditional if statement. Use of continue statement, change the normal sequence of execution of statements inside loop.
Syntax of continue Statement
Control Flow of continue Statement
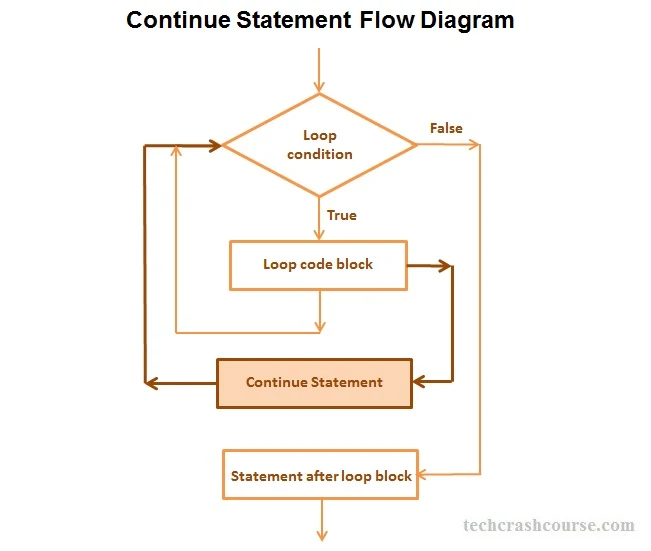
If we are using continue statement inside nested loop, then it will only skip statements of inner loop from where continue is executed.
C Program to show use of continue statement
#include <stdio.h> int main(){ /* * This program calculate sum of even numbers */ int N, counter, sum=0; printf("Enter a positive number\n"); scanf("%d", &N); for(counter=1; counter <= N; counter++){ /* * Using continue statement to skip odd numbers */ if(counter%2 == 1){ continue; } sum+= counter; } printf("Sum of even numbers between 1 to %d = %d", N, sum); return(0); }Above program find sum of all even numbers between 1 to N, using for loop. It counter is odd number then continue statement will skip the sum statement.
Output
Enter a positive number 6 Sum of even numbers between 1 to 6 = 12
Best Practices for Using the Continue Statement in C
- Use Continue Judiciously : Exercise caution while employing the use of the "continue" function. Although the continuing statement might be advantageous in some situations, it should be employed with discretion. Excessive utilization of the "continue" statement might result in intricate and less comprehensible code logic. Evaluate if the utilization of the "continue" statement improves or impedes the comprehensibility of your code.
- Clearly Document Intention :When employing the "continue" statement within a loop, it is advisable to clearly articulate your aim by including explanatory comments. This practice enhances the comprehensibility of the code for other writers. The continue statement is utilized to skip the remaining code inside a loop iteration and proceed to the next iteration. It plays a crucial role in the logical flow of the loop by allowing some iterations to be bypassed based on specific conditions.
- Avoid Unnecessary Nesting : In certain instances, the reasoning that necessitates the utilization of the "continue" statement might be reorganized to obviate its necessity. Explore the possibility of employing alternate conditions or loop designs that accomplish the identical result without resorting to the use of continue.
- Consider Alternative Logic : In some cases, the logic that leads to the use of continue can be restructured to eliminate the need for it. Consider using alternative conditions or loop constructs that achieve the same result without the use of continue.
- Check Loop Conditions : When using continue within a loop, ensure that the loop's condition is correctly evaluated after the continue statement. This is particularly important in cases where the loop condition involves multiple variables or complex expressions.