A switch statement allows a variable or value of an expression to be tested for equality against a list of possible case values and when match is found, the block of code associated with that case is executed.
The switch statement is a powerful control flow construct in C programming that provides an efficient way to handle multiple conditions based on the value of an expression. It is particularly useful when you have a single variable with multiple possible values, and you want to execute different blocks of code based on the value of that variable.
The Syntax of the Switch Statement
The switch statement has a straightforward syntax that consists of a switch keyword, an expression (usually a variable), and a series of case labels. The basic structure is as follows
switch (expression) { case constant1: // Code to be executed if expression equals constant1 break; case constant2: // Code to be executed if expression equals constant2 break; // ... default: // Code to be executed if expression does not // match any constant }
- expression : The variable or expression whose value is compared with the constants.
- constant1, constant2, ... : Possible values that the expression might match.
- break : A keyword that terminates the switch statement. If omitted, the control falls through to the next case.
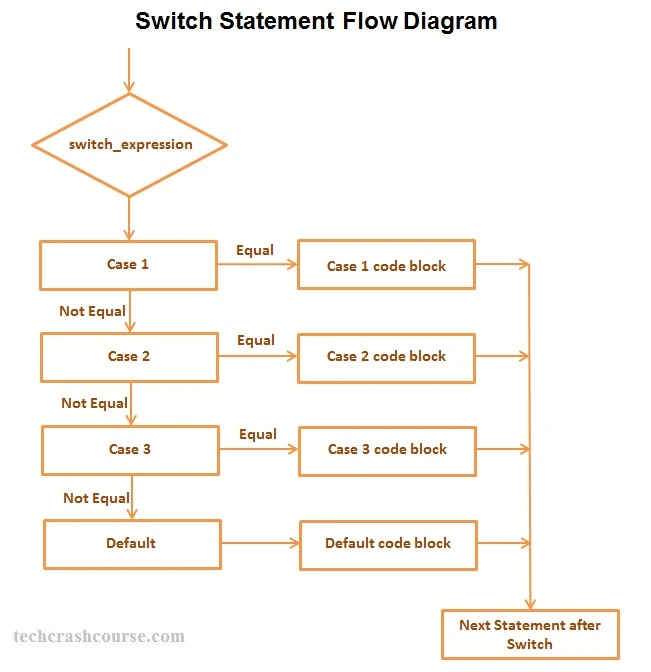
Important Points about Switch Statement
- Switch case performs equality check of the value of expression/variable against the list of case values.
- The expression in switch case must evaluates to return an integer, character or enumerated type.
- You can use any number of case statements within a switch. The expression value is compared with the constant after case.
- The data type of the value of expression/variable must be same as the data type of case constants.
- The break statement is optional.The break statement at the end of each case cause switch statement to exit. If break statement is not used, all statements below that case statement are also executed until it found a break statement.
The Role of the Default Case
The default case in a switch statement serves as the "catch-all" block that gets executed when none of the case values match the expression. While the default case is optional, including it is generally considered good practice to handle unexpected values.
Without a default case, if the expression does not match any of the specified constants, the control will simply exit the switch statement. Including a default case allows you to provide meaningful feedback or perform specific actions when unexpected values are encountered.
C Program to print grade of a student using Switch case statement
#include <stdio.h> int main(){ int marks; printf("Enter your marks between 0 to 100\n"); scanf("%d", &marks); switch(marks/10){ case 10 : case 9 : /* Marks between 90-100 */ printf("Your Grade : A\n" ); break; case 8 : case 7 : /* Marks between 70-89 */ printf("Your Grade : B\n" ); break; case 6 : /* Marks between 60-69 */ printf("Your Grade : C\n" ); break; case 5 : case 4 : /* Marks between 40-59 */ printf("Your Grade : D\n" ); break; default : /* Marks less than 40 */ printf("You failed\n" ); } return 0; }Above program check whether a student passed or failed in examination using if statement.
Output
Enter your marks between 0 to 100 55 Your Grade : D
Enter your marks between 0 to 100 99 Your Grade : A
Enter your marks between 0 to 100 30 You failed
Using Switch with Enums
The switch statement works particularly well with enumerated types (enum). Enums provide a way to create named integer constants, making code more readable and maintainable.
#include <stdio.h> enum Days { MON, TUE, WED, THU, FRI, SAT, SUN }; int main() { enum Days day = WED; switch (day) { case MON: printf("Monday\n"); break; case TUE: printf("Tuesday\n"); break; case WED: printf("Wednesday\n"); break; // ... default: printf("Invalid day\n"); } return 0; }In this example, the enum Days defines constants for each day of the week. The switch statement then uses these constants as case values.
Best Practices of Using Switch Statement in C
- Use Switch for Equality Checks : Switch statements are well for equality checks against several constants. Use if-else expressions for more sophisticated conditions.
- Keep Switches Simple : Write brief switch statements. Switches that are too lengthy may indicate that the logic should be refactored into smaller functions.
- Include a Default Case : Include a default case to handle unexpected values. It gently handles expressions that do not match any constants.
switch (day) { case MON: // Code break; case TUE: // Code break; // ... default: // Code for handling unexpected values }
- Use Enums for Readability : nums increase code readability when working with related constants. Enums give values meaningful names, making programming clearer.
enum Days { MON, TUE, WED, THU, FRI, SAT, SUN };
- Use Break Appropriately : Use break statements to run only the intended code block. Each case block should end with break unless fall-through is needed.
switch (grade) { case 'A': // Code break; case 'B': // Code break; // ... } // Avoid fall-through behavior switch (day) { case MON: // Code (no break) case TUE: // Code break; }
Potential Pitfalls of Using Switch Statement
- Forgetting Break Statements : One common mistake is forgetting to include break statements at the end of each case block. Without break, the control will fall through to the next case, and subsequent code blocks will be executed.
switch (day) { case MON: printf("Monday\n"); case TUE: printf("Tuesday\n"); break; // ... }
To avoid this, ensure that each case block ends with break unless fall-through behavior is intentionally desired. - Missing Default Case : Neglecting to include a default case can result in unexpected behavior when the expression does not match any of the specified constants. Always include a default case to handle unexpected values gracefully.
switch (day) { case MON: // Code break; case TUE: // Code break; // ... default: // Code for handling unexpected values }
- Using Non-Integral Types : The expression within a switch statement must evaluate to an integral or enumerated type. Using non-integral types or floating-point numbers will result in a compilation error.
switch (floatingPointVar) { // Error: Switch expression of floating-point type }
Ensure that the expression is of an appropriate type for use in a switch statement.
Drawbacks of Switch Case
We cannot do conditional tests using relational or logical operators like \, >, \=, &&, ||, etc.; switch statements may only be used for equality comparisons.
Conclusion
When managing several situations depending on the value of an expression, the switch-case statement in C is a useful tool. A neat and effective method to organize your code is to use the switch-case statement, whether you're working with enums or numeric numbers. You may write legible and maintainable code by following best practices, which include utilizing enums for constants, making each case straightforward, and offering a default case. For every C developer, knowing the subtleties of fall-through, carefully testing your switch statements, and deciding whether to use switch instead of if-else if are vital.