The if else ladder statement in C programming language is used to test set of conditions in sequence. An if condition is tested only when all previous if conditions in if-else ladder is false. If any of the conditional expression evaluates to true, then it will execute the corresponding code block and exits whole if-else ladder.
Syntax of if else ladder statement
statement1;
} else if (condition_expression_Two) {
statement2;
} else if (condition_expression_Three) {
statement3;
} else {
statement4;
}
- First of all condition_expression_One is tested and if it is true then statement1 will be executed and control comes out out of whole if else ladder.
- If condition_expression_One is false then only condition_expression_Two is tested. Control will keep on flowing downward, If none of the conditional expression is true.
- The last else is the default block of code which will gets executed if none of the conditional expression is true.
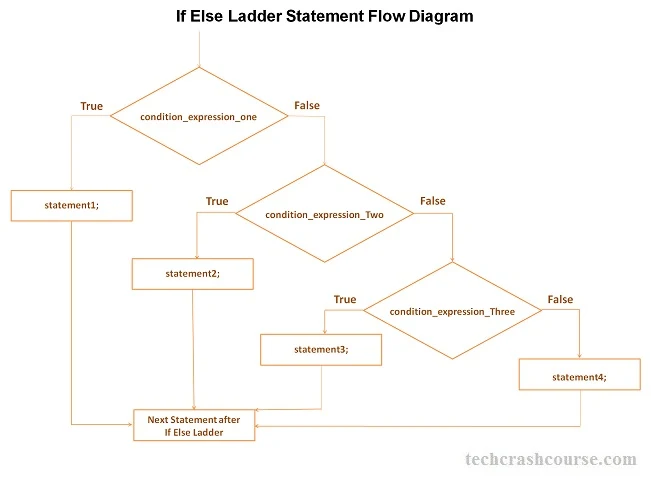
C Program to print grade of a student using If Else Ladder Statement
#include<stdio.h> int main(){ int marks; printf("Enter your marks between 0-100\n"); scanf("%d", &marks); /* Using if else ladder statement to print Grade of a Student */ if(marks >= 90){ /* Marks between 90-100 */ printf("YOUR GRADE : A\n"); } else if (marks >= 70 && marks < 90){ /* Marks between 70-89 */ printf("YOUR GRADE : B\n"); } else if (marks >= 50 && marks < 70){ /* Marks between 50-69 */ printf("YOUR GRADE : C\n"); } else { /* Marks less than 50 */ printf("YOUR GRADE : Failed\n"); } return(0); }Above program check whether a student passed or failed in examination using if statement.
Output
Enter your marks 96 YOUR GRADE : A
Enter your marks 75 YOUR GRADE : B
Enter your marks 60 YOUR GRADE : C
Enter your marks 35 YOUR GRADE : Failed
- The condition_expression is a boolean expression. It must evaluate to true or false value(In C, all non-zero and non-null values are considered as true and zero and null value is considered as false).
- We may use more than one condition inside if else ladder statement
For Example:
if(condition_one && condition_two) {
/* if code block */
} else if(condition_three) {
/* else code block */
} - Default else block is optional in if-else ladder statement.
- Opening and Closing Braces are optional, If the block of code of if else statement contains only one statement.
For Example:
if(condition_expression)
statement1;
else if(condition_expression)
statement2;
In above example only statement1 will gets executed if condition_expression is true.
Nested If Statement in C
The nested if statement in C programming language is used when multiple conditions need to be tested. The inner statement will execute only when outer if statement is true otherwise control won't even reach inner if statement.
Syntax of Nested If Statement
/* Code to be executed when condition_expression_one is true */
statement1;
if(condition_expression_two) {
/* Code to be executed when condition_expression_one and
condition_expression_two both are true */
statement2;
}
}
If the condition_expression_one is true, then statement1 is executed and inner if statements condition_expression_two is tested. If condition_expression_two is also true then only statement 2 will get executed.
C Program to show use of Nested If Statements to test multiple conditions
#include<stdio.h> int main(){ int num; printf("Enter a numbers\n"); scanf("%d", &num); /* Using nested if statement to check two conditions*/ /* Outer if statement */ if(num < 500){ printf("First Condition is true\n"); if(num > 100){ printf("First and Second conditions are true\n"); } } printf("This will print always\n"); return(0); }Output
Enter a numbers 80 First Condition is true This will print always
Enter a numbers 200 First Condition is true First and Second conditions are true This will print always
Enter a numbers 800 This will print always
Best practices of if-else ladders and nested if statements
- Limit Nesting Depth : To avoid excessive nesting of if statements, prevent the complexity of the code. Code that is overly nested may be more difficult to read and more prone to containing logical errors.
- Use Descriptive Variable Names : It is advisable to select descriptive variable names that effectively communicate the intended function of the conditions under assessment. By doing so, the code becomes more transparent and requires fewer superfluous comments.
- Consider Switch Statements : One potential trade-off between a lengthy if-else cascade and a switch statement is the readability of the former, particularly when comparing the value of a single variable to multiple constant values.
switch (dayOfWeek) { case 1: // Sunday break; case 2: // Monday break; // ... default: // Invalid day }
- Use Parentheses for Clarity : For the sake of clarity, enclose nested if statements in parentheses to specify the extent of each condition. This practice aids in mitigating perplexity and improves the readability of the code.
if (condition1) { if (condition2 || condition3) { // Code } } else { // Code }
- Break Down Complex Conditions : If a condition becomes too complex, consider breaking it down into simpler conditions and assigning the results to boolean variables. This can improve code readability.
int isPositive = (num > 0); int isEven = (num % 2 == 0); if (isPositive) { // Code if (isEven) { // Code } else { // Code } } else { // Code }
- Align Code for Readability : Ensure that code blocks contained within if-else ladders and nested if statements have consistent alignment and indentation. This improves code readability and clarifies the code's structure.
if (condition1) { // Code block } else if (condition2) { // Code block } else { // Code block }
- Use Else-if Sparingly : Although else-if statements can be beneficial, their application should be considered cautious. For greater clarity, when conditions are mutually exclusive, it is advisable to employ distinct if statements.
if (condition1) { // Code } else if (condition2) { // Code } else if (condition3) { // Code } else { // Code }