Here is a C program to find the area and perimeter of a trapezium(trapezoid). A trapezium is a quadrilateral with at least one pair of parallel sides, other two sides may not be parallel. The parallel sides are called the bases of the trapezium and the other two sides are called it's legs. The perpendicular distance between parallel sides is called height of trapezium.
In North America, A trapezium is referred to as a trapezoid.
- The sum of the two adjacent angles of a trapezium is 180 degrees.
- A pair of opposite sides of a trapezium are parallel.
- As a pair of sides are parallel. Hence, The angle between a side and diagonal is equal to the angle between the opposite parallel side and the diagonal.
- The cosines(cos) of two adjacent angles sum to 0. As adjacent angles are supplementary.
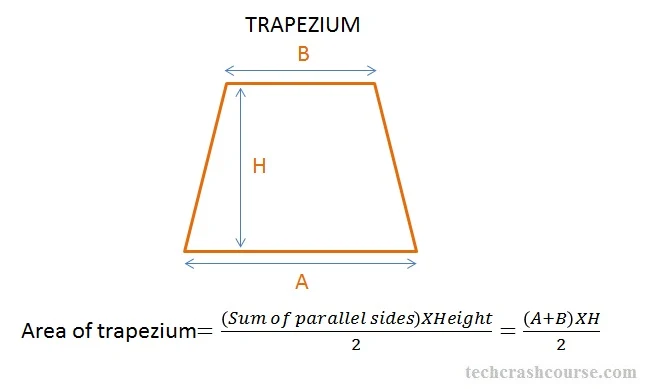
Types of Trapezium
- Right Trapezium : Two adjacent internal angles of a trapezium are 90 degrees.
- Scalene Trapezium : All sides of a trapezium are of different length.
- Isosceles Trapezium : The length of two legs of a trapezium are same and the base angles have the same measure. It is also known as acute Trapezium.
- All types of parallelograms are also Trapezium, like rectangles, squares and rhombuses.
The area of a trapezium is the amount of two-dimensional space inside it's boundary. In other words, the area of trapezoid can be calculated by placing the trapezoid over a grid and counting the number of square units it takes to completely it.
- Area of Trapezium = 1/2 X (A + B) X H
H is perpendicular distance between the parallel sides, it is know as height of trapezoid.
The perimeter of trapezoid is the total distance around the outer boundary. We can find the perimeter of trapezium by adding the length of all sides.
- Perimeter of Trapezium = Sum of all sides of Trapezium = A + B + C + D
C Program to find the area of the trapezium
#include <stdio.h> #include <math.h> int main(){ float parallelSideOne, parallelSideTwo, height, area; printf("Enter the length of parallel sides of trapezium\n"); scanf("%f %f", ¶llelSideOne, ¶llelSideTwo); printf("Enter the height of trapezium\n"); scanf("%f", &height); area = 1.0/2 * (parallelSideOne+parallelSideTwo) * height; printf("Area of trapezium : %0.4f\n", area); return 0; }Output
Enter the length of parallel sides of trapezium 4 7 Enter the height of trapezium 3.5 Area of trapezium : 19.2500
Above program first takes length of parallel sides and height as input form user using scanf function and stores it in three floating point variables. Then it calculates the area of trapezium using the formula given above. Then, it prints the area of trapezoid on screen using printf function.
C Program to find the perimeter of the trapezium
#include <stdio.h> #include <math.h> int main(){ float sideOne, sideTwo, sideThree, sideFour, perimeter; printf("Enter the length sides of trapezium\n"); scanf("%f %f %f %f", &sideOne, &sideTwo, &sideThree, &sideFour); perimeter = sideOne + sideTwo + sideThree + sideFour; printf("Perimeter of trapezium : %0.4f\n", perimeter); return 0; }Output
Enter the length of parallel sides of trapezium 4 7 Enter the length sides of trapezium 2 3 4 5 Perimeter of trapezium : 14.0000
Above program first takes length of all sides of trapezium as input form user using scanf function and stores it in four floating point variables sideOne, sideTwo, sideThree and sideFour. Then it calculates the perimeter of trapezium using the formula given above. Then, it prints the perimeter of trapezium on screen.