A switch statement in C++ allows a variable or value of an expression to be tested for equality against a list of possible case values and when match is found, the block of code associated with that case is executed. It is similar to if..else ladder statement, but the syntax of switch statement is more readable and easy to understand.
The switch-case statement in C++ is a control flow mechanism designed to handle multiple conditions based on the value of an expression. It provides an efficient and structured way to execute different blocks of code depending on the value of a variable. Think of it as a multi-destination roadmap for your program.
Syntax of Switch Statement
The basic syntax of a switch-case statement looks like this:switch (expression) { case value1: // Code to be executed if expression equals value1 break; case value2: // Code to be executed if expression equals value2 break; // ... More cases default: // Code to be executed if none of the cases match }The expression is evaluated once, and its value is compared against the values specified in the case labels. If a match is found, the corresponding block of code is executed.
The Break Statement
Each case block is terminated by the break statement. The break statement exits the switch statement, preventing fall-through to subsequent cases. Without it, the code would continue to execute the subsequent cases until a break or the end of the switch statement is reached.
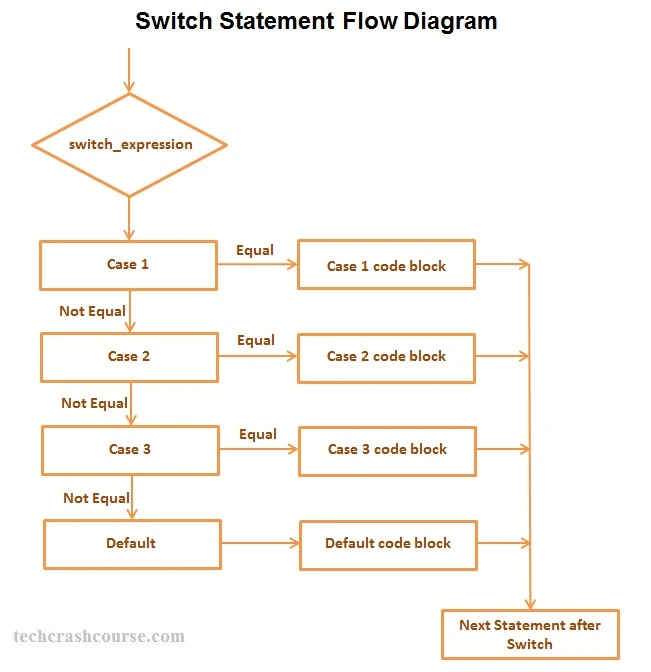
Advantages and Disadvantages of Switch Case in C++
- The complexity of the program increases as the number of constants to compare increases. Switch statement provides a way to write easy to manage. Can be more efficient than a series of if-else if statements, especially for a large number of cases. The switch statement is often optimized by the compiler for faster execution.
- Switch statement provides a default case handler when no case matches.
- We can nest switch statements like if else statements.
- Provides a clear and concise way to handle multiple conditions. Enums or named constants can enhance code readability.
- The switch-case statement is designed for simple equality comparisons. It cannot handle more complex conditions or expressions, limiting its expressiveness compared to if-else statements.
- Unlike some other programming languages, C++ switch-case statements do not support ranges or inequalities. This limitation can lead to verbose and less readable code when dealing with non-contiguous values.
- Cases in a switch statement are restricted to constant integral values or enumerated types. This limitation means you cannot use variables or non-integer expressions as case labels.
- The fall-through behavior, where execution continues to the next case after a match, can lead to unintended bugs if not handled carefully. While fall-through can be intentional and useful in some cases, it often requires explicit comments to convey the programmer's intent.
- Unlike if-else statements, switch-case statements cannot directly return a value. The use of the break statement to exit the switch block makes it challenging to return a value from a function within the switch statement.
- The structure of a switch-case statement can make it challenging to debug, especially when dealing with fall-through cases or complex logic within cases. The lack of direct support for runtime evaluation and dynamic labels can also hinder debugging efforts.
- Unlike some modern programming languages, C++ switch-case statements cannot be used for string comparisons. If you need to compare strings, you must resort to if-else statements or other techniques.
C++ Switch Statement Example Program
#include <iostream> using namespace std; int main(){ int n; cout << "Enter a number between 1 to 6\n"; cin >> n; switch(n){ case 1 : /* following code will execute when n == 1 */ cout << "ONE\n"; break; case 2 : /* following code will execute when n == 2 */ cout << "TWO\n"; break; case 3 : /* following code will execute when n == 3 */ cout << "THREE\n"; break; case 4 : case 5 : case 6 : /* following code will execute when n==4 or n==5 or n==6*/ cout << "FOUR, FIVE OR SIX\n"; break; default : /* following code will execute when n < 1 or n > 6 */ cout << "INVALID INPUT\n"; } cout << "Always gets printed\n"; return 0; }
Output
Enter a number between 1 to 6 3 THREE Always gets printed
Enter a number between 1 to 6 6 FOUR, FIVE OR SIX Always gets printed
Enter a number between 1 to 6 8 INVALID INPUT Always gets printed
Using Enums with Switch-Case
Enums (enumerations) in C++ provide a way to define named constant values. Using enums with the switch-case statement can make your code more readable, as it replaces numeric values with meaningful names.
#include <iostream> enum Day { Monday,Tuesday,Wednesday,Thursday,Friday,Saturday,Sunday }; int main() { Day today = Wednesday; switch (today) { case Monday: std::cout << "It's the start of the week!" << std::endl; break; case Wednesday: std::cout << "It's hump day!" << std::endl; break; // ... Continue for the rest of the days default: std::cout << "Invalid day" << std::endl; } return 0; }This way, the code is more expressive, and you're less likely to mix up numeric values.
Important Points about Switch Statement in C++
- Switch case performs only equality check of the value of expression/variable against the list of case values.
- Case constants must be unique. We cannot write two case blocks with same constants.
- The expression in switch case must evaluates to return an integer, character or enumerated type.
- You can use any number of case statements within a switch.
- One switch statement can have maximum of one default label. Default block can be placed anywhere in switch statement.
- The data type of the value of expression must be same as the data type of case constants.
- The break statement is optional. The break statement at the end of each case cause switch statement to exit. If break statement is not used, all statements below that case statement are also executed until it found a break statement.
- The default code block gets executed when none of the case matches with expression. default case is optional and doesn't require a break statement.
Best Practices for Using Switch-Case Statements in C++
- Always Include a Default Case : Include a default case to handle unexpected values and provide meaningful error messages.
- Use Enums for Clarity : If applicable, use enums or named constants to make your code more readable.
- Avoid Fall-Through Unless Intentional : Be cautious with fall-through; use comments to indicate intentional fall-through if necessary.
- Keep Switch Statements Concise : If a switch statement becomes too long, consider refactoring your code or using alternative approaches.
- Avoid Complex Expression : Keep case expressions simple to enhance code readability.
Conclusion
You've successfully navigated the seas of the switch-case statement in C++. You now possess the knowledge to make informed decisions, handle multiple conditions, and enhance the structure and efficiency of your code.
As you continue your coding voyage, remember that the switch-case statement is a versatile tool in your toolkit. Use it wisely, and it will guide your program through the waves of decision-making with grace and precision.