If..else statement in java first evaluates conditional expression. If conditional expression evaluates to true then if block gets executed otherwise else block gets executed.
Unlike if statement, if..else statement provides a alternate path of execution whenever conditional expression evaluates to false. Only either if block or else block
can get executed at a time not both.
If else statement is used when we want to execute different set of statements depending upon whether certain condition is true of false. In this comprehensive tutorial, we will explore the syntax, usage, and best practices associated with the if-else statement in Java.
Syntax of if..else statement
The if-else statement in Java follows a simple syntax:if(condition_expression) { statements; } else { statements; }The condition_expression is a boolean expression that determines whether the block of code inside the if statement or the else statement should be executed.

Java if else statement program
public class IfElseExample { public static void main(String[] args) { int number = 10; if (number > 5) { System.out.println("The number is greater than 5."); } else { System.out.println("The number is not greater than 5."); } } }Output
10 is EvenIn this example, the program checks whether the number variable is greater than 5. If true, it executes the code inside the if block; otherwise, it executes the code inside the else block.
Nested If-Else Statements
You can nest if-else statements to create more complex decision-making structures. This allows you to check multiple conditions and execute different code blocks accordingly.
public class NestedIfElseExample { public static void main(String[] args) { int num = 15; if (num > 10) { System.out.println("Number is greater than 10."); if (num % 2 == 0) { System.out.println("Number is even."); } else { System.out.println("Number is odd."); } } else { System.out.println("Number is not greater than 10."); } } }Here, the outer if-else statement checks if num is greater than 10. If true, it enters the block and evaluates another condition using a nested if-else statement to determine if the number is even or odd.
If..Else Ladder Statement in Java
If..else ladder statement in java is used when we want to test multiple conditions and execute different code blocks for different conditions. After the if block we can add multiple if..else block to check multiple conditions.
Syntax of if-else ladder statement
if(condition_expression_One) { statements; } else if (condition_expression_Two) { statements; } else if (condition_expression_Three) { statements; } else { statements; }
It evaluates the conditions in sequence, from top to bottom. An else-if condition is evaluated only if all previous conditions in ladder evaluated false. If any of the conditional expression evaluates to true, then it will execute the corresponding code block and exits whole if-else ladder statement.
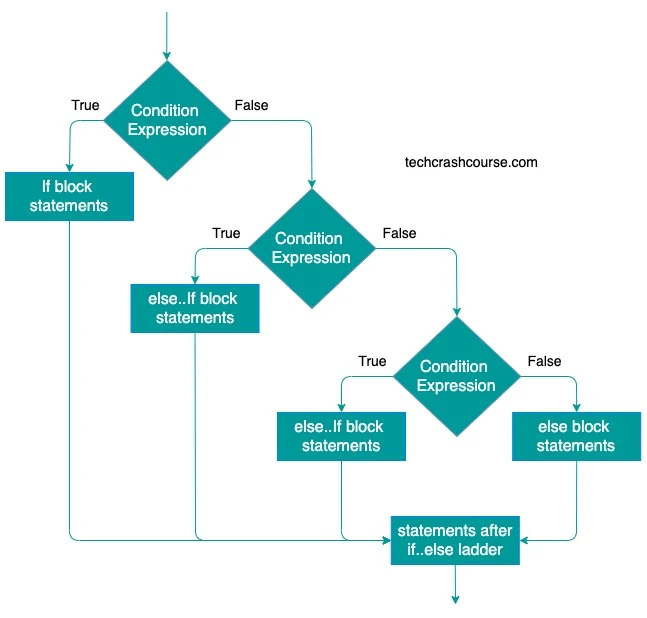
Java if else ladder statement program
public class IfElseLadderStatement { public static void main(String[] args) { int marks = 90; if (marks < 40) { System.out.println("FAILED"); } else if (marks >= 40 && marks < 60) { System.out.println("GRADE C"); } else if (marks >= 60 && marks < 80) { System.out.println("GRADE B"); } else { System.out.println("GRADE A"); } } }Output
GRADE A
Ternary Operator as a Concise If-Else
Java provides the ternary operator (? :) as a concise way to express simple conditional statements. It is a shorthand form of an if-else statement.
variable = (condition) ? expression_if_true : expression_if_false;
public class TernaryOperatorExample { public static void main(String[] args) { int x = 10; int y = 20; int max = (x > y) ? x : y; System.out.println("The maximum value is: " + max); } }In this example, the ternary operator is used to determine the maximum value between x and y and assign it to the variable max.
Best Practices of Using If-Else Statements
- Use Clear and Descriptive Conditions : When writing conditions, prioritize clarity and readability. Use meaningful variable names and avoid overly complex expressions. Clear conditions make your code more understandable to others and to your future self.
- Avoid Nested If Statements if Possible : While nesting if-else statements is sometimes necessary, excessive nesting can make code harder to read. Aim for a balance between nesting and readability. Consider refactoring deeply nested structures into separate methods or using alternative control structures.
- Use Switch for Multiple Conditions : If you're checking multiple values of a single variable, consider using a switch statement. It's a cleaner and more readable alternative to a series of if-else if conditions.
- Keep Code DRY (Don't Repeat Yourself) : Avoid repeating similar conditions across your code. If you find yourself using the same condition in multiple places, encapsulate the logic in a method. This promotes code reuse and makes updates easier.
- Handle Edge Cases : Ensure that your if-else statements cover all possible scenarios, including edge cases and unexpected inputs. Think about the range of values your variables can take and handle each case explicitly.
- Test Your Code : Before deploying your code, perform thorough testing with different inputs to verify that your if-else statements behave as expected. Automated testing frameworks can be particularly useful in this regard.
Conclusion
Mastering the if-else statement is crucial for Java developers to create logic that responds dynamically to different conditions. Understanding its syntax, applying best practices, and incorporating additional control structures like switch and the ternary operator will empower you to write efficient, readable, and maintainable code. As you gain experience, you'll find that the if-else statement is a versatile tool for building robust Java applications.