C programming language supports multi dimensional Arrays. A multi-dimensional array in C is essentially an array of arrays. While one-dimensional arrays are like a single row or column, multi-dimensional arrays add additional dimensions, forming a grid-like structure. Commonly used multi-dimensional arrays include two-dimensional arrays (matrices) and three-dimensional arrays.
- Multi dimensional arrays have more than one subscript variables.
- Multi dimensional array is also called as matrix.
- Multi dimensional arrays are array of arrays.
Declaration of Multi Dimensional Array
Above statement will declare an array of N dimensions of name array_name, where each element of array is of type data_type. The maximum number of elements that can be stored in a multi dimensional array array_name is size1 X size2 X size3...sixeN.
For Example:Declaration of two dimensional integer array
int board[8][8];
Declaration of three dimensional character array
char cube[50][60][30];
Two Dimensional Array in C
Two dimensional arrays are most common type of multi dimensional array. A two dimensional array in C language is represented in the form 2D matrix having rows and columns.
- A two dimensional matrix is an array of one dimensional arrays.
- Two dimensional array requires two subscript variables or indexes.
- One subscript represents the row index while other represent column index of an element in matrix.
- Elements of a two dimensional array are stored in contiguous memory location. First all elements of first row are store then all elements of second row and so on.
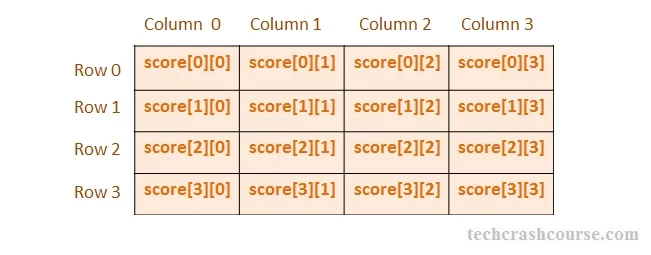
Any element in two dimensional matrix is uniquely identified by array_name[row_Index][column_Index], where row_index and column_index are the subscripts that uniquely specifies the position of an element in a 2D matrix.
An element in second row and third column of an two dimensional matrix whose name is board is accessed by board[1][2].
Like single dimensional array, indexing starts from 0 instead of 1.
We can declare a two dimensional matrix of R rows and C columns as follows:
data_type array_name[R][C];For Example:
A two dimensional integer matrix of 3 rows and 3 columns can be declared as
int score[3][3];
Initialization of Two Dimensional Array
Two dimensional arrays can be initialized by specifying elements for each row inside curly braces. The following declaration initializes a two dimensional matrix of 4 rows and 3 columns.
int matrix[4][3] = { {1, 2, 3}, /* Initialization of first row */ {4, 5, 6}, /* Initialization of second row */ {7, 8, 9}, /* Initialization of third row */ {10, 11, 12}, /* Initialization of fourth row */ };Similarly, we can initialize any multi dimensional array.
A two dimensional matrix can be initialized without using any internal curly braces as follows:
int matrix[4][3] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12};
In above declaration, compiler will assign first four values(1, 2, 3 and 4) to first row of matrix and within a row it will populate elements from left to right. Then next four elements to second row and so on.
C program to find sum of all elements of two dimensional array
#include <stdio.h> int main(){ /* Two dimentional array declaration */ int matrix[20][20]; int rowCounter, colCounter, rows, cols, sum=0; printf("Enter size of a matrix\n"); scanf("%d %d", &rows, &cols); /* Populating elements inside matrix */ printf("Enter elements of a matrix of size %dX%d\n", rows, cols); for(rowCounter = 0; rowCounter < rows; rowCounter++){ for(colCounter = 0; colCounter < cols; colCounter++){ scanf("%d", &matrix[rowCounter][colCounter]); } } /* Accessing all elements of matrix to calculate their sum */ for(rowCounter = 0; rowCounter < rows; rowCounter++){ for(colCounter = 0; colCounter < cols; colCounter++){ sum += matrix[rowCounter][colCounter]; } } printf("Sum of all elements = %d", sum); return 0; }Above program first declares a matrix of size 20X20 then populates it by taking input form user. Now using two for loops it access each element of matrix and add their values to a variable sum.
Output
Enter size of a matrix 3 3 Enter elements of a matrix of size 3X3 1 2 3 4 5 6 7 8 9 Sum of all elements = 45
Three-Dimensional Arrays in C
A three-dimensional array adds another dimension, creating a cube-like structure. To declare a three-dimensional array, you specify the number of layers, rows, and columns:
#include <stdio.h> int main() { // Declaration and initialization of a 3D array int threeDimArray[2][3][4] = { { {1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12} }, { {13, 14, 15, 16}, {17, 18, 19, 20}, {21, 22, 23, 24} } }; // Accessing elements of the 3D array printf("Element at layer 2, row 1, column 3: %d\n", threeDimArray[1][0][2]); // Output: 15 return 0; }
In this example, threeDimArray is a 2x3x4 three-dimensional array, and the element at layer 2, row 1, column 3 is accessed and printed.
Best Practices of Using Multi Dimensional Arrays in C
- Use Descriptive Variable Names : Choose meaningful and descriptive names for your multi-dimensional arrays. Provide information about the purpose or contents of the array to improve code readability.
// Poor naming int arr[3][4]; // Improved naming int studentScores[3][4];
Descriptive names make your code more self-explanatory, reducing the need for extensive comments. - Avoid Magic Numbers : Avoid using "magic numbers" (hard-coded constants) when declaring the size of multi-dimensional arrays. Define constants or use sizeof to determine array sizes dynamically.
// Magic numbers int scores[5][3]; // Improved approach #define NUM_STUDENTS 5 #define NUM_SUBJECTS 3 int scores[NUM_STUDENTS][NUM_SUBJECTS];
Using constants improves code maintainability and allows for easy adjustments. - Initialize Arrays Explicitly : Explicitly initialize multi-dimensional arrays, especially when declaring them. Avoid relying on default values to ensure predictable behavior.
// Explicit initialization int matrix[3][3] = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} };
Explicit initialization provides clarity and avoids potential bugs caused by relying on default values. - Avoid Excessive Nesting : Limit the depth of array nesting to maintain code readability. Excessive nesting can lead to confusion and make the code harder to understand.
// Excessive nesting int cube[2][3][4][5]; // Prefer flatter structures int flatMatrix[2][15];
Flatter structures are generally easier to work with and comprehend. - Check Array Bounds : Always perform boundary checks when accessing elements in a multi-dimensional array. Ensure that indices are within the declared bounds to prevent buffer overflows.
// Boundary check if (row < NUM_ROWS && col < NUM_COLUMNS) { // Access array element } else { // Handle out-of-bounds error
Boundary checks are essential for preventing undefined behavior and improving program robustness.
Conclusion
To work with multidimensional arrays in C effectively, you need to follow good practices, be clear, and know how memory is laid out. If you follow these best practices, you can write code that is not only correct and useful, but also easy to read, keep up to date, and work on with other people. These tips will help you make strong and expandable C programs, whether you are working with matrices, tables, or more complicated data structures.