The for loop in C programming language is used to execute a code block several times until the given condition is true. The for loop is mainly used to perform repetitive tasks.
Syntax of for Loop
/* code to be executed */
}
for(i = 1; i <= 100; i++){ printf("%d", i); }Above for loop prints integers from 1 to 100;
For Loop Syntax Description
- Initialization : The Initialization statement allows us to declare and initialize any loop variables. It is executed only once at the beginning of the for loop.
- Condition : After initialization, condition expression is evaluated. It is a boolean expression which decides whether to go inside the loop and iterate or terminate for loop. if condition expression evaluates to true, the code block inside for loop gets executed otherwise it will terminate for loop and control goes to the next statement after the for loop.
- Update : After code block of for loop gets executed, control comes back to update statements. It allow us to modify any loop variable for next iteration of loop.
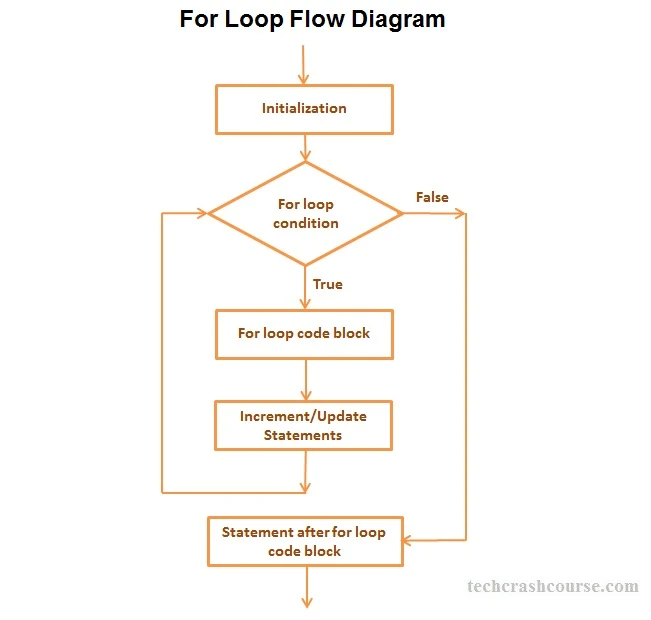
- The Initialization statement will be executed first. We can declare and initialize any number of loop control variables here.
- After the Initialization statement the condition expression is evaluated. If the value of condition expression is true then code block of for loop will be executed otherwise the loop will be terminated.
- After execution of the code block of for loop control goes to update statements of the loop statement which modifies the loop control variables.
- After modifying control variables in update statements, control again goes to condition expression.
- For loop iteration continues unless condition expression becomes false or for loop gets terminated using break statement.
C Program to show use of for Loop
#include<stdio.h> int main(){ int N, counter, sum=0; printf("Enter a positive number\n"); scanf("%d", &N); /* Using for loop to find the sum of all integers from 1 to N */ for(counter=1; counter <= N; counter++){ sum+= counter; } printf("Sum of Integers from 1 to %d = %d", N, sum); return(0); }Above program finds sum of all integers from 1 to N, using for loop.
Output
Enter a positive number 7 Sum of Integers from 1 to 7 = 28
- The condition_expression in for loop is a boolean expression. It must evaluate to true or false value(In C, all non-zero and non-null values are considered as true and zero and null value is considered as false).
- Initialization statement, Condition expression and Update statement are all optional in for loop.
- You can use multiple initialization statements and update statements inside for loop.
For Example:
for(i=0, j=50; i < 100; i++, j--) - Opening and Closing braces are not required for single statement inside for loop code block.
For Example:
for(i = 0; i < 100; i++)
sum+= i; - We can also use infinite for loops like for(;;), which will never terminate. You should add terminating condition using break statement in order to terminate infinite for loop.
For Example:
for(;;){
if(.....){
break;
}
}
Applications of the For Loop in C
- Iterating Over Arrays : Iterating over an array's elements is a popular usage for the for loop. This lets you work with each element individually or examine the contents of the array.
#include <stdio.h> int main() { int numbers[] = {1, 2, 3, 4, 5}; // Print each element of the array for (int i = 0; i < 5; i++) { printf("%d ", numbers[i]); } return 0; }
In this example, the for loop iterates over the elements of the numbers array, printing each element. - Counting and Summation : Counting and summation operations are frequently performed using the for loop. For instance, adding up all of the elements in an array or counting the instances of a certain element in an array.
#include <stdio.h> int main() { int numbers[] = {1, 2, 3, 4, 5}; int sum = 0; // Sum all elements of the array for (int i = 0; i < 5; i++) { sum += numbers[i]; } printf("Sum: %d\n", sum); return 0; }
In this example, the numbers array's elements are iterated over by the for loop, which adds each element to the sum variable. - Generating Patterns : Another handy application of the for loop is the creation of numerical sequences or patterns. This frequently occurs when printing a triangle of stars or numbers, for example.
#include <stdio.h> int main() { // Print a right-angled triangle of stars for (int i = 1; i <= 5; i++) { for (int j = 1; j <= i; j++) { printf("* "); } printf("\n"); } return 0; }
In this example, there are nested for loops to create a right-angled triangle pattern of stars.
Best Practices of Using the For Loop in C
- Initialize Loop Control Variable Properly : Initialize Control Variable Loop Consistently ensure that the loop control variable is initialized within the for loop preamble. This aids in mitigating unforeseen behavior and guarantees that the variable commences with a predetermined value.
// Avoid int i; for (i = 0; i < 5; i++) { // Code } // Preferred for (int i = 0; i < 5; i++) { // Code }
By specifying the loop control variable in the loop preamble, one restricts its applicability to the loop itself, thereby mitigating the potential for inadvertent alteration in other sections of the code. - Be Mindful of Loop Termination Conditions : It is crucial to exercise caution regarding loop termination conditions in order to prevent the occurrence of potentially infinite loops. Ensure that the condition will ultimately return false in order to avert the program from becoming entangled in an infinite loop.
// Potential infinite loop for (int i = 0; i > -1; i++) { // Code }
The condition i > -1 will invariably hold true in this particular instance, resulting in an infinite loop. Exercise caution when applying conditions that, under certain conditions, may not be satisfied. - Use Meaningful Loop Control Variable Names : To improve the readibility of code, select names for loop control variables that are meaningful. This is especially crucial when working with nested loops, as variables with obvious names facilitate code comprehension.
// Less readable for (int i = 0; i < 5; i++) { for (int j = 0; j < 3; j++) { // Code } } // More readable for (int row = 0; row < 5; row++) { for (int col = 0; col < 3; col++) { // Code } }
Use of row and col as names for loop control variables in this example enhances code clarity. - Keep the Loop Body Concise : It is essential that the code contained within the body of the for loop be succinct and centered on the intended purpose of the loop. To enhance legibility and maintainability, contemplate decomposing the loop body into distinct functions or loops if it becomes excessively intricate.
// Less readable for (int i = 0; i < 5; i++) { // Complex code } // More readable for (int i = 0; i < 5; i++) { // Separate complex code into functions simpleFunction(); }
Complex duties can be decomposed into functions to increase the readability of the for loop and the modularity of the code. - Test the Loop with Different Inputs : Conduct Extensive Testing of the Loop with Diverse Inputs: Strictly examine the for loop using a variety of inputs, incorporating extreme cases and boundary conditions. This facilitates the loop's adherence to expectations and its ability to handle any conceivable scenario.
for (int i = 0; i <= 100; i += 10) { // Code }
In this example, testing the loop with different step sizes and ranges ensures its correctness under various conditions.
Infinite For Loops
Infinite loops can occur if the loop termination condition is not properly defined or if the loop control variable is not updated correctly.
// Infinite loop for (int i = 0; i >= 0; i++) { // Code }In this example, the loop control variable i is decremented, but the loop termination condition is always true, leading to an infinite loop.
Conclusion
C programming's for loop is strong and adaptable, making repetitive operations efficient. The for loop lets you develop clean, simple, and dependable code by grasping its syntax, applications, and best practices.
As you learn C programming, practice using the for loop, try different loop control variable updates, and pay attention to loop termination circumstances. It will help you grasp the for loop and write efficient, error-free loops in your programs.