Array in C programming language is a collection of fixed size data belongings to the same data type. An array is a data structure which can store a number of variables of same data type in sequence. These similar elements could be of type int, float, double, char etc.
For Exampleint age[100]; /* Integer array of size 100 */ float salary[10]; /* floating point array of size 10 */
Suppose, you want to store salary of 100 employees. You can store salaries of all employees by creating 100 variable of int data type individually like employeeSalary1, employeeSalary2, employeeSalary3 .... employeeSalary100. This is very tedious, time consuming and difficult to maintain method of storing 100 salaries.
C programming language provides support for array data structure, which solves the problem of storing N similar data. We can declare an integer array of length 100 to store salaries of 100 employees
int employeeSalary[100];
In the above array declaration, we declared an array of name "employeeSalary" which can store maximum of 100 integer data in contiguous memory locations. We can access the individual elements of an array using their index.
- employeeSalary[0] refers to the first element of array.
- employeeSalary[1] refers to the second element of array.
- employeeSalary[99] refers to the last element of array which is 100th element.
Suppose, we have an integer array of size 7 whose name is score. The base address or starting address of array score is 1000(base address is same as address of first element score[0]) and the size of int be 2 bytes. Then, the address of second element(score[1]) will be 1002, address of third element(score[2]) will be 1004 and so on.
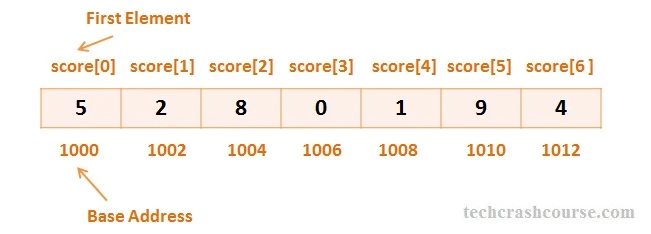
Important Facts about Arrays in C
- An array is a collection of variables of same data types.
- All elements of array are stored in the contiguous memory locations.
- The size of array must be a constant integral value.
- Individual elements in an array can be accessed by the name of the array and an integer enclosed in square bracket called subscript/index variable like employeeSalary[5].
- Array is a random access data structure. you can access any element of array in just one statement.
- The first element in an array is at index 0, whereas the last element is at index (size_of_array - 1).
- Less amount of code : Using array we can aggregate N variables of same data type in a single data structure. Otherwise we have to declare N individual variables.
- Easy access of elements : We can access any element of array using array name and index. We can access all elements serially by iterating from index 0 to size-1 using a loop.
- Easy to implement algorithms : Certain algorithms can be easily implemented using array like searching and sorting, finding maximum and minimum elements.
- Random Access : We can access any elements of array in O(1) time complexity.
Declaration of Array in C
Like any other variable in C, we must declare an array first before using it. Below is the syntax of declaring a one-dimensional array.
- array_name : It is a valid C identifier representing the name of the array.
- array_size : It is the maximum number of elements which can be stored in array. It must be an integer constant greater than zero.
- data_type : It represents the data type of the elements to be stored in array.
- An Array of 10 Integers
int marks[10]; - An Array of 50 Characters.
char name[50]; - Two Dimensional Array of Integers.
int matrix[10][10];
Types of Array in C
There are two types of Arrays in C
- One Dimensional Array
- Multi Dimensional Array
- Single dimensional array is used to store data in sequential order.
- Single dimensional array contains only one subscript/index in their declaration, like
int marks[100]; - In one dimensional array, we can access any element using one index reference, like marks[5].
- Array having more than one subscript variable/index is called multi dimensional array.
- Multi dimensional array is array of array.
- Multi dimensional array contains multiple subscript/index in their declaration, like
int matrix[10][20]; - In multi dimensional array, we can access any element using multiple index reference, like matrix[4][8].
Initialization of Array in C
Initialization of Array in C programming language. Arrays in C programming language is not initialized automatically at the time of declaration. By default, array elements contain garbage value. Like any other variable in C, we can initialize arrays at the time of declaration.
Array Initialization by Specifying Array Sizeint score[7] = {5,2,9,1,1,4,7};Initialization of array after declaration.
int score[7]; score[0] = 5; score[1] = 2; score[2] = 9; score[3] = 1; score[4] = 1; score[5] = 4; score[6] = 7;Array Initialization Without Specifying Array Size
int score[] = {5,2,9,1,1,4,7};
In above declaration, compiler counts the number Of elements inside curly braces{} and determines the size of array score. Then, compiler will create an array of size 7 and initialize it with value provided between curly braces{} as discussed above.
C Program to show Array Initialization
#include <stdio.h> int main(){ /* Array initialization by specifying array size */ int roll[7] = {1,2,3,4,5,6,7}; /* Array initialization by without specifying array size */ int marks[] = {5,2,9,1,1,4,7}; int i; /* Printing array elements using loop */ printf("Roll_Number Marks\n"); for(i = 0; i < 7; i++){ printf("%5d %9d\n", roll[i], marks[i]); } return 0; }Output
Roll_Number Marks 1 5 2 2 3 9 4 1 5 1 6 4 7 7
Accessing Array Elements of C
We can access any array element using array name and subscript/index written inside pair of square brackets [].
For Example:Suppose we have an integer array of length 5 whose name is marks.
int marks[5] = {5,2,9,1,1};Now we can access elements of array marks using subscript followed by array name.
- marks[0] = First element of array marks = 5
- marks[1] = Second element of array marks = 2
- marks[2] = Third element of array marks = 9
- marks[3] = Fourth element of array marks = 1
- marks[4] = Last element of array marks = 1
C Program to print all Array Elements
#include <stdio.h> int main(){ int value[7] = {1,2,3,4,5,6,7}; int i; /* Printing array elements using loop */ for(i = 0; i < 7; i++){ printf("Element at index %d is %d\n", i, value[i]); } return 0; }Output
Element at index 0 is 1 Element at index 1 is 2 Element at index 2 is 3 Element at index 3 is 4 Element at index 4 is 5 Element at index 5 is 6 Element at index 6 is 7
Passing Array to Function in C
How to pass array to a function in C ?
In C programming, an array element or whole array can be passed to a function like any other basic data type. We can pass an array of any dimension to a function as argument. Passing an array to a function uses pass by reference, which means any change in array data inside will reflect globally.
Passing Single Element of an Array to Function
We can pass one element of an array to a function like passing any other basic data type variable.
C program to pass one element of an array to function
#include <stdio.h> int getSquare(int num){ return num*num; } int main(){ int array[5]={1, 2, 3, 4, 5}; int counter;; for(counter = 0; counter < 5; counter++){ /* Passing individual array elements to function */ printf("Square of %d is %d\n", array[counter], getSquare(array[counter])); } return 0; }Output
Square of 1 is 1 Square of 2 is 4 Square of 3 is 9 Square of 4 is 16 Square of 5 is 25
Passing one dimensional array to function
We can pass a one dimensional array to a function by passing the base address(address of first element of an array) of the array. We can either pas the name of the array(which is equivalent to base address) or pass the address of first element of array like &array[0]. Similarly, we can pass multi dimensional array also as formal parameters.
Different ways of declaring function which takes an array as input.- Function argument as a pointer to the data type of array.
int testFunction(int *array){ /* Function body */ }
- By specifying size of an array in function parameters.
int testFunction(int array[10]){ /* Function body */ }
- By passing unsized array in function parameters.
int testFunction(int array[]){ /* Function body */ }
C Program to Pass One Dimensional Array as Parameter to a Function
#include <stdio.h> /* This function takes integer pointer as input */ void printArrayOne(int *array, int size){ int i; for(i=0; i<size; i++){ printf("%d ", array[i]); } printf("\n"); } /* This function takes sized array as input */ void printArrayTwo(int array[5], int size){ int i; for(i=0; i<size; i++){ printf("%d ", array[i]); } printf("\n"); } /* This function takes unsized array as input */ void printArrayThree(int array[], int size){ int i; for(i=0; i<size; i++){ printf("%d ", array[i]); } printf("\n"); } int main(){ int array[5]={1, 2, 3, 4, 5}; printArrayOne(array, 5); printArrayTwo(array, 5); printArrayThree(array, 5); return 0; }Output
1 2 3 4 5 1 2 3 4 5 1 2 3 4 5
Returning Array from a Function in C
We can return a pointer to the base address(address of first element of array) of array from a function like any basic data type. However, C programming language does not allow to return whole array from a function.
We should not return base pointer of a local array declared inside a function because as soon as control returns from a function all local variables gets destroyed. If we want to return a local array then we should declare it as a static variable so that it retains it's value after function call.
C Program to Return an Array from a Function
#include <stdio.h> /* This function returns an array of N even numbers */ int* getEvenNumbers(int N){ /* Declaration of a static local integer array */ static int evenNumberArray[100]; int i, even = 2; for(i=0; i<N; i++){ evenNumberArray[i] = even; even += 2; } /* Returning base address of evenNumberArray array*/ return evenNumberArray; } int main(){ int *array, counter; array = getEvenNumbers(10); printf("Even Numbers\n"); for(counter=0; counter<10; counter++){ printf("%d\n", array[counter]); } return 0; }Output
Even Numbers 2 4 6 8 10 12 14 16 18 20