Here is a C program to find the area and circumference of a circle. A circle is a simple geometrical shape. A circle is a set of all points in a 2D plane that are at a given distance from a given point called centre. A circle can be uniquely identified by it's center co-ordinates and radius.
- Center of a Circle is a point inside the circle and is at an equal distance from all of the points on its circumference.
- Radius is the length of the a segment joining the centre of the circle to any point on the circle.
- Diameter is the length of the a segment passing through centre of the circle and joining two points on edge. Diameter is twice of Radius.
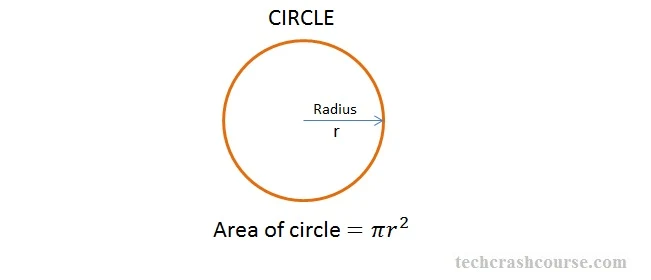
The area of circle is the amount of two-dimensional space taken up by a circle. The area of a circle can be calculated by placing the circle over a grid and counting the
number of squares that circle covers. Different shapes have different ways to find the area.
We can compute the area of a Circle if you know its radius.
Where PI is a constant which is equal to 22/7 or 3.141(approx)
Area is measured in square units.
Circumference is the linear distance around the edge of a circle. It is the length of the curved line which defines the boundary of a circle. The perimeter of a circle is called the circumference.
- Circumference or Circle = 2 X PI X Radius
- Circumference or Circle = PI X Diameter
C Program to find the area of the circle
#include <stdio.h> #define PI 3.141 int main(){ float radius, area; printf("Enter radius of circle\n"); scanf("%f", &radius); area = PI*radius*radius; printf("Area of circle : %0.4f\n", area); return 0; }In above program, we first take radius of a circle as an input from user using scanf function and store it in floating point variable named "radius". Now, we calculate the area of circle(PI X radius X radius) and store it in variable area. Then we print the area of circle on screen using printf function.
Output
Enter radius of circle 5 Area of circle : 78.5250
C Program to find the area of a circle using pow function
We can use pow function of math.h header file to calculate Radius^2(Radius square) instead of multiplying Radius with itself. double pow(double a, double b) returns a raised to the power of b (a^b). Below program uses pow function to calculate the area of circle.
#include <stdio.h> #include <math.h> #define PI 3.141 int main(){ float radius, area; printf("Enter radius of circle\n"); scanf("%f", &radius); area = PI*pow(radius, 2); printf("Area of circle : %0.4f\n", area); return 0; }
Output
Enter radius of circle 3 Area of circle : 28.2690