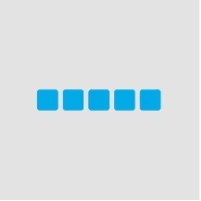
Array Data Structure
An Array is a data structure that stores a collection of elements of the same data type. The elements of an array are stored in contiguous memory locations, and each element can be accessed using an index. The index of the first element in an array is 0, and the index of the last element is one less than the size of the array. A
Arrays are fundamental data structures in computer science that provide a systematic way to store and organize elements of the same data type in contiguous memory locations. They offer constant-time access to elements, making them efficient for tasks like retrieval and manipulation. Arrays are used to store data in a way that makes it easy to access and manipulate the data.

Why Array Data Structure ?
Arrays are useful when we need to store a collection of values of the same data type. They allow us to access individual elements quickly and easily, and to perform operations on entire collections of data in a single operation. Arrays are widely used in programming for a variety of applications, such as sorting, searching, and data manipulation. The size of an array is fixed upon declaration, and all elements must be of the same data type. Key characteristics of arrays include:
- Indexing : Elements are accessed by their position in the array, starting from index 0 for the first element.
- Contiguous Memory : Elements are stored in adjacent memory locations, facilitating quick access.
- Fixed Size : The size of the array is determined at the time of declaration and cannot be changed during runtime.
- Homogeneous Elements : All elements must be of the same data type.
Advantages and Limitations of Arrays
Advantages of Arrays
- Arrays provide fast and efficient access to individual elements.
- Arrays are straightforward and efficient for tasks like traversal and sorting.
- Arrays are widely supported in programming languages and are easy to use.
- Arrays use contiguous memory, minimizing memory overhead.
Limitations of Arrays
- Arrays have a fixed size, which means that we cannot add or remove elements easily.
- They require contiguous memory allocation, which can be a limitation in some situations.
- Array elements must be of the same data type.
- Inserting or deleting elements in the middle of an array can be inefficient.
How to Declare an Array
To declare an array in most programming languages, we specify the type of data that the array will contain, followed by its name, and then the size of the array in brackets. For example, to declare an array of integers in C with a size of 10, we can use the following syntax:
int myArray[10];
This declares an array called myArray that can hold 10 integers. The size of the array must be specified when the array is declared, and it cannot be changed later.
How to Initialize an Array
We can initialize an array at the time of declaration by specifying a list of values in curly braces. For example, to initialize an array of integers with the values 1, 2, 3, 4, and 5 in C, we can use the following syntax:
int myArray[] = {1, 2, 3, 4, 5};
This initializes an array called myArray with the values 1, 2, 3, 4, and 5. The size of the array is automatically set to the number of values in the list.
How to Access Array Elements
We can access individual elements of an array by specifying their index or position in the array. In most programming languages, the index of the first element in an array is 0. For example, to access the second element of an array of integers called myArray in C, we can use the following syntax:
int secondElement = myArray[1];
This retrieves the second element of the myArray array and assigns it to a variable called secondElement. The index of the element we want to access is enclosed in square brackets.
Array Program in C
Here is an example program that demonstrates how to declare, initialize, and access elements of an array in C.
#include <stdio.h> int main() { // Declare and initialize an array of integers int myArray[] = {5, 10, 15, 20, 25}; // Access individual elements of the array // and print them to the console printf("First element: %d\n", myArray[0]); printf("Second element: %d\n", myArray[1]); printf("Third element: %d\n", myArray[2]); printf("Fourth element: %d\n", myArray[3]); printf("Fifth element: %d\n", myArray[4]); return 0; }Output
First element: 5 Second element: 10 Third element: 15 Fourth element: 20 Fifth element: 25
In this example, we declare an array of integers called myArray with five elements and initialize it with the values 5, 10, 15, 20, and 25. We then access each element of the array using its index and print it to the console using printf.
Best Practices of Using Array Data Structures
- Understand the nature of your data and the operations you need to perform. While arrays are suitable for certain tasks, other data structures like linked lists, sets, or maps may be more appropriate for specific scenarios. Consider the complexity of operations (insertions, deletions, lookups) and choose a data structure that aligns with the requirements of your application.
- Declare the size of the array based on the maximum number of elements it needs to accommodate. Avoid using excessively large arrays that may lead to unnecessary memory consumption. Consider dynamic arrays or resizable collections if the size of the data is not known in advance or may change during runtime.
- In languages that support dynamic arrays (e.g., ArrayList in Java or Vector in C++), consider using them when the size of the data is expected to change dynamically. Dynamic arrays automatically handle resizing and provide flexibility without the need to predefine a fixed size.
- Be mindful of memory usage, especially in resource-constrained environments. Avoid creating excessively large arrays that may lead to inefficient memory utilization. Release memory when it is no longer needed to prevent memory leaks.
- Initialize arrays with default values to ensure that each element has a known initial state. Be cautious when initializing large arrays to avoid unnecessary computation or resource consumption.
- When traversing arrays, prefer using the appropriate loop constructs provided by the programming language (e.g., for loop) for clarity and efficiency. Leverage parallel processing or vectorization for large-scale data processing to enhance traversal speed.
- Use built-in sorting functions provided by the programming language (e.g., Arrays.sort() in Java) for efficiency and correctness. Employ optimized searching algorithms (e.g., binary search for sorted arrays) when searching for specific elements.
- Be vigilant about array indices to prevent buffer overflows or underflows. Ensure that index values are within the valid range (0 to length-1). Check array bounds before accessing or modifying elements to avoid runtime errors.
- Adopt clear and consistent naming conventions for arrays to enhance code readability. Choose meaningful names that convey the purpose and contents of the array. Use plural forms or descriptive names to indicate the array's role in the context.
Conclusion
In conclusion, arrays are fundamental data structures that provide efficient ways to organize, access, and manipulate data. Understanding their characteristics, operations, and use cases is crucial for every programmer. Whether dealing with simple lists or multidimensional structures, arrays are powerful tools that form the basis for many other data structures and algorithms in computer science.