A Java method is a collection of statements that are grouped together to perform a specific task. Methods in Java allow us to reuse the code without retyping the code again and again. In this tutorial, we will learn about syntax of a method, types of methods, method declaration, and how to call a method in Java.
- A Java program is a set of methods calling each other.
- We can use any number of methods in a Java program.
- All Java programs must contains at least one main() method.
Method Declaration in Java
Here is an example method to find sum of two integers with description of individual component of a method syntax.
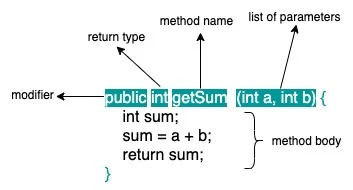
- modifier : It defines the access type of the method, that is from where it can be called in your program. There are four types of access specifiers in java public, private, protected and default.
- return type : The data type of the value returned by the method. If a method doesn't return any value then its return type is void.
- method name : It is an identifier that is used to refer to the particular method in a program.
- list of parameters : This segment defines the type, order, and number of parameters of a method which you want to pass to a method. We can pass any number of arguments to a method. If there are no parameters, you must use empty parentheses ().
- method body : It includes the set of statements that needs to be executed to perform some specific task. The method body is enclosed inside the curly braces {}. Method body much return some value unless return type of method is void.
Advantages of Using Methods
- Code Reusability : Methods allow you to define functionality once and reuse it multiple times throughout your program, promoting code reusability.
- Readability : By breaking down complex logic into smaller methods, your code becomes more readable and easier to comprehend.
- Maintenance : Methods simplify the maintenance of code. If a change is required, you only need to update the method rather than making changes in multiple places.
- Abstraction : Methods provide a level of abstraction, allowing you to focus on high-level functionality without worrying about the details of the implementation.
Types of Methods in Java
There are two types of methods in Java.
Predefined Method
In Java, predefined methods are the method that is already defined in the Java class libraries. It is also known as the standard library method or built-in method. We can directly call these methods in our program at any point. Examples of pre-defined methods are abs(), ceil(), length(), equals(), compareTo(), toString() etc. These methods are already defined in the java libraries.
User-defined Method
The method written by the programmer is known as a user-defined method. These methods were defined as per the specific requirement of the program.
Calling a Method in Java
After writing a method in Java, we have to call this method to perform the task defined inside method body. Here's is how we can call the previously define "getSum" method.
getSum(5, 10);
When a method A calls another method B, program control is transferred to the method B. The statements inside method B executes to perform specific task and when method B terminates either by a return statement or when its method body's execution finished, program control returns back to the calling method A.

Java program to call a method
public class CallingMethod { public static int getSum(int a, int b) { int sum; sum = a + b; System.out.println("Inside getSum"); return sum; } public static void main(String[] args) { int i = 5, j = 10; // Calling getSum method int total = getSum(i, j); System.out.println("Total= " + total); } }Output
Inside getSum Total= 15
In the above program, we have created a method called getSum(). The method takes two parameters a and b and returns sum of a and b.
int total = object.getSum(i, j);We called getSum method by passing two int arguments. We stored the returned value of method getSum in variable total.
Returning Values from Methods
- Return Statement : The return statement is used to exit a method and optionally return a value to the caller. If a method has a return type other than void, it must include a return statement with the value of that type.
// Method with a return type int add(int a, int b) { return a + b; }
In this example, the add method returns the sum of two integers. The return statement specifies the value that the method will provide to the caller. - Void Methods : Methods with a return type of void do not return a value. They perform a task or operation without providing any result.
// Void method void displayMessage(String message) { System.out.println(message); }
In this example, the displayMessage method takes a String parameter and prints it without returning any value. - Returning Objects : A method can also return an object, allowing you to encapsulate related data and behavior within a single entity.
// Method that returns an object Person createPerson(String name, int age) { return new Person(name, age); }
In this example, the createPerson method returns a new Person object with the specified name and age.
Memory Allocation for Methods Calls
Methods calls are implemented through a stack sata structure. Whenever a method is called a stack entry is created within the stack frame and after that, the method arguments, local variables and value to be returned by the method are stored in this stack frame. After the completion of method execution the allocated stack frame will get deleted.
That's why a local variable defined within method body cannot be accessed after method execution finished.
Advantages of Methods in Java
- We can call a method any number of times in a program from any place which avoid rewriting same code again and again.
- A large Java program can be divide into set of methods, where each method owns the responsibility of performing one specific task.
- Splitting a Java program into methods, makes it easy to maintain and makes the code more modular.
- We can create a utility library containing commonly used methods, so that it can be reused by other programs also.
Best Practices of Using Array Data Structures
- Descriptive Method Names : Choose descriptive names for your methods that clearly indicate their purpose. This enhances code readability and makes it easier for others (and your future self) to understand your code.
- Keep Methods Small and Focused : Aim for small, focused methods that perform a single task. This promotes code modularity and makes it easier to test and maintain your code.
- Use Parameters Effectively : Avoid using too many parameters in a method. If a method requires a large number of parameters, consider using an object to encapsulate related parameters.
- Document Your Methods : Provide meaningful comments or documentation for your methods. This helps other developers understand how to use the method and what it is designed to accomplish.
Conclusion
In this tutorial, we've covered the basics of methods in Java, including their syntax, types, parameters, return values, and best practices. Understanding how to create and use methods is essential for writing clean, modular, and maintainable Java code. As you continue your Java programming journey, practice implementing methods in different scenarios to solidify your understanding and improve your coding skills.