The super keyword in Java is a reference variable used to refer to the immediate parent class object. It is primarily used in the context of inheritance to distinguish between the members (fields and methods) of the subclass and those of the superclass. The super keyword is essential for invoking superclass methods, accessing superclass fields, and calling the superclass constructor.
It is used to refer to the immediate parent class object, providing a means to access its members, invoke its methods, and facilitate the creation and functioning of subclasses. In this comprehensive tutorial, we will delve into the various applications of the super keyword, explore its syntax, and discuss best practices for its effective use.
For Example, Let's say class A extends class B. Whenever you create an object of A, then "this" will have reference of object of A whereas parent class object is referenced by "super".
Syntax of the super Keyword
- To Call Superclass Method
super.methodName();
- To Access Superclass Field
super.fieldName;
- To Call Superclass Constructor
super(arguments);
- super is used to call constructor of immediate super class.
- super is used to access instance variables of immediate super class.
- super is used to call methods of immediate super class.
Let’s understand above mentioned uses of super keywprd one by one.
Using super to call constructor of super class
We can use super() in the constructor of subclass to call the constructor of parent class. It's a special form of the super keyword. We can call parameterized constructor of parent class by passing appropriate argument while calling super class constructor.
- If we don't call super class's constructor from base class explicitly, then compiler automatically ads a default constructor of super class in every constructor of subclass.
- super must be the first statement inside the constructor of subclass.
- When we create an object of subclass then constructor of super class gets called first then constructor of subclass.
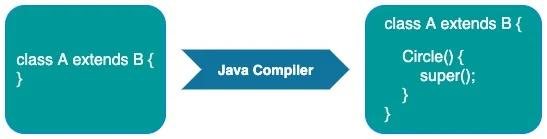
Syntax
- super(); is used to call the no-argument constructor of super class.
- super(...); is used to call parametrized constructor of super class.
class Fruit { String color; Fruit() { System.out.println("Fruit No-Arg Constructor"); } Fruit(String color) { this.color = color; System.out.println("Color :" + color); } } class Apple extends Fruit { String name; Apple() { System.out.println("Apple No-Arg Constructor"); } Apple(String color, String name) { super(color); this.name = name; System.out.println("Name :" + name); } } public class SuperConstructor { public static void main(String[] args) { // Using No-arg Constructor Apple apple1 = new Apple(); // Using parameterized Constructor Apple apple2 = new Apple("Red", "Gala"); } }Output
Fruit No-Arg Constructor Apple No-Arg Constructor Color :Red Name :Gala
In above program, we created a super class called "Fruit" containing on member variable color. Fruit class have one No-arg constructor and one parameterized constructor. Next, we created a classs called "Apple"(subclass) which extends Fruit class(super class).
- Object apple1 of Apple class is created by calling No-Arg constructor of Apple, it calls the No-Arg constructor of Fruit class. The default or no-arg construtor of subclass will automatically calls the default or no-arg constructor of super class.
- Object apple2 of Apple class is created by calling the parameterized constructor of Apple. It explicitly called the parameterized constructor of Fruit using super(color) as the first statement of Apple's constructor. Compiler will not call parameterized constructor of super class from parameterized constructor of subclass automatically.
Using super to access instance variable of parent class
We can use super to access the instance variables of immediate parent class. It is useful when parent and child class have fields with same name and we want to access field of parent class from subclass. If we don't use super reference in subclass then JVM will always refer to subclass variable and base class variable will be hidden from subclass.
class Fruit { String name; Fruit() { name = "Apple"; } } class Apple extends Fruit { String name; Apple() { name = "Gala"; } public void printDetails() { System.out.println(super.name + " Fruit"); System.out.println(name + " Apple"); } } public class SuperClassVariable { public static void main(String[] args) { Apple apple1 = new Apple(); apple1.printDetails(); } }Output
Apple Fruit Gala Apple
In above program, we have defined the same instance variable "name" in both the superclass Fruit and the subclass Apple. We create an object apple1 of Apple class by calling no-arg constructor or Apple. Then we called printDetails method of apple1 object.
Inside printDetails method "super.name" refers to name variable of super class whereas "name" refers to sub class variable.
Using super to invoke instance method of parent class
We can use super to access overridden method of parent class. Sometimes, we override few methods of parent class in subclass as a result we have to use super reference in subclass to call overridden method of parent class to avoid ambiguity.
If a parent class method is not overridden in subclass then we do not need to use super to call parent class method.
class Person { public void print() { System.out.println("Inside Person"); } } class Employee extends Person { public void print() { System.out.println("Inside Employee"); } public void printDetails() { print(); super.print(); } } public class SuperClassMethod { public static void main(String[] args) { Employee emp = new Employee(); emp.printDetails(); } }Output
Inside Employee Inside Person
In above program, we have defined the same instance method "print" in both superclass Person and subclass Employee. We create an object emp of Employee class by calling default constructor of Employee.
Since print method is defined in both the classes, the print method of subclass Employee overrides the print method of superclass Person. Which mean, if you call print method of emp object, it will invoke print method of Employee class instead of print method of Person class.
To call the print method of super class using subclass object we need to use super.print().
Best Practices for Using 'super'
- Use super Selectively : While the super keyword is a valuable tool, it should be used selectively and when necessary. In many cases, proper class design and polymorphism can reduce the need for explicit use of super.
- Clarify Code Intent : When using super, it's a good practice to do so explicitly to clarify the code's intent. This makes it clear that you are intentionally referencing a superclass member or invoking a superclass constructor.
- Be Mindful of Shadowing : When a subclass declares a field or method with the same name as a field or method in the superclass, it is said to shadow the superclass member. Be mindful of shadowing and use super to differentiate between the superclass and subclass members.
- Follow Constructor Chaining Rules : When calling constructors using super(), ensure that the super() call or the this() call (if any) is the first statement in the constructor. Constructor chaining is crucial for initializing the state of the object correctly.
- Leverage Polymorphism : In many scenarios, polymorphism can be a more elegant solution than explicit use of super. Design your classes to take advantage of polymorphism, allowing for flexibility and extensibility.
Conclusion
The super keyword in Java is a versatile tool that facilitates effective communication between a subclass and its immediate parent class. Whether used to invoke superclass methods, access superclass fields, or call superclass constructors, super plays a crucial role in object-oriented programming and inheritance. By understanding its syntax, applications, and best practices, Java developers can harness the power of super to create well-designed, maintainable, and extensible code. Whether you're a novice or an experienced developer, mastering the super keyword is an essential skill in the Java programming language.