We are going to learn a simple java program to print "Hello World" string on screen. Printing "hello world" string program is traditional first program for any new programmer
while learning a new programming language.
This program will give us a brief overview of how to write and compile a java program and about the fundamental structure of a java program.
To write your first java program, you will need
- The Java Development Kit (JDK).
- A text editor (like notepad ) or IDE (like Eclipse).
Setting Up Your Java Environment
- Install Java Development Kit (JDK) : Visit the official Oracle website or adopt OpenJDK to download the latest version of the JDK suitable for your operating system. Follow the installation instructions provided for your specific platform (Windows, macOS, or Linux).
- Set Up Environment Variables : Once the JDK is installed, set up the JAVA_HOME environment variable to point to the JDK installation directory. Additionally, add the bin directory to the system's PATH variable.
- Verify Installation : Open a terminal or command prompt and type java -version and javac -version to verify that the JDK installation was successful.
Java Program to Print "Hello World" String
Here is the java program to print "Hello World" string on screen.
package com.tcc.java.tutorial; /* First Java program to print "Hello World" String on screen */ public class HelloWorld{ public static void main(String args[]){ // prints Hello World System.out.println("Hello World"); } }Output
Hello World
How to Write and Compile a Java Program
-
Write
- Open a text editor and type the above mentioned java program to print "Hello World".
- Save this file as HelloWorld.java
-
Compile
- Open command prompt and go to your current working directory where you saved your HelloWorld.java file. Let's say you saved it as C:\programs.
- Compile your code by typing "javac HelloWorld.java" in command prompt. Your program will compile successfully, If your program doesn't contain any syntax error.
-
Execute
- Now, execute your java program by typing "java HelloWorld" in command prompt.
-
Output
- You will see "Hello World" printed on your console.
Understanding Hello World Java Program
Let's analyse above program line by line.
-
/* First Java program to print "Hello World" String on screen */
This is a multiline comment in java, similar to the multi-line comment in C++. They start with /* and end with */. Whatever is written in between them is a comment. These comments will be ignored by the compiler at compilation time. -
public class HelloWorld{
Public is an access specifier, class is a java jeyword used for declaring a class and HelloWorld is the name is class. Name of class name must exactly match the name of program file. Don't worry, if you don't understand anything here, we will discuss about it in detail on later tutorials. -
public static void main(String args[]){
Public is an access specifier, which means accessible by all. static is a java keyword, a static function can be called directly without creating object. void is the return type of main method. String args[] is the command line argument of main function. -
// prints Hello World
It is a single line comment. It starts with "//" and ends with a newline character. Everything on a line after // is ignored by a compiler -
System.out.println("Hello World");
It is used to print a string on screen in a separate line.
How Java Programming Language Work
We can classify the working of a Java program in three steps
- First of all, we need to write a java program and save it as a .java file.
- Second, we need to use a compiler to compiles java program. At compile time, a Java compiler(javac, which is present in JDK) converts Java source code(HelloWorld.java) into bytecode. The bytecode gets saved as a .class file by the compiler itself. The Java bytecode is a redesigned version of the java source code and this bytecode can be executed anywhere irrespective of the machine on which it has been built. This makes java platform independent.
- At last, JVM interpret this bytecode and reads all the statements step by step which will further convert it to the machine-level language so that the machine can execute the code.
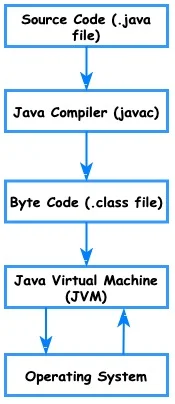
- A multiline comment starts with /* and ends with */ .
- The name of the program file must match with the name of class.
- Every java program must have one main() function from where program execution starts.
Common Errors and Troubleshooting
- "javac is not recognized as an internal or external command" (Windows) : This error indicates that the javac command is not in the system's PATH. Double-check your JDK installation and ensure that the bin directory is added to the PATH variable.
- "Error: Could not find or load main class HelloWorld" : This error occurs when the Java Virtual Machine (JVM) cannot find the specified class. Ensure that the file name and class name match exactly, including capitalization.
- Syntax Errors : If you encounter syntax errors during compilation, carefully review your code and look for typos or incorrect syntax. The error messages provided by the compiler are often helpful in identifying the issue.
Welcome to the Java World!
With your first "Hello, World!" program, you've taken the initial steps into the vast world of Java programming. As you continue your journey, don't hesitate to explore, experiment, and challenge yourself with increasingly complex projects. The skills you develop in Java will not only serve as a foundation for other programming languages but also open doors to a wide range of applications, from web development to mobile app development and enterprise-level systems. Happy coding!