A constructor is a special method inside a class which is invoked to create an object of that class. We can also write custom constructors to initialize an object.
Not just initialization, you can do lots of things inside a constructor like calling another method, opening a file, updating a database etc.
Whenever an object is created using the new keyword, at least one constructor of the class is called.
If there is no constructor defined inside the class body then Java compiler automatically generates a default constructor for that class.
Syntax of Java Constructor
The constructor syntax is close to the method syntax but unlike any regular member method, a constructor method syntax is following restrictions:
- The name of the constructor method should be same as the name of class.
- There is no return type of constructor.
- A constructor cannot be final, native, abstract, static, strictfp or synchronized.
Here is an example of No-Arg constructors of a class
class ClassName { ClassName() { // constructor body } }Here, ClassName() is a constructor for class ClassName. It has the same name as that of the class and doesn't have a return type.
Why Use Constructors ?
- Initialization : Constructors initialize the attributes of an object, providing initial values to the object's state.
- Default Values : Constructors can set default values for attributes if no values are provided during object creation.
- Complex Initialization : For complex objects, constructors can perform intricate setup tasks or connect to external resources.
Difference between Constructor and Method
Constructor | Method |
---|---|
Constructors are used to initialize an object. | Member methods are used to implement object behaviors and provide an interface for other classes. |
These is no return type for Constructors. | Member method must have return type. |
Constructor is called automatically. | Member methods don't gets called automatically. |
If we don't write a constructor then java compiler will create a default constructor. | Java compiler don't generate member methods automatically. |
The name of constructor must be same as the class name. | Member Method's name can be any valid java identifier not necessarily class name. |
Types of Constructors
Java support three types of constructor methods
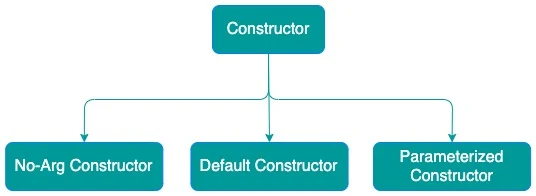
Default constructor
Defining a constructor method is optional in java. If we don't define any constructor for a class then java compiler will automatically create a constructor method without any
argument. This constructor is known as default constructor.

class Circle { long radius; } public class DefaultConstructor { public static void main(String[] args) { // Creating an object of Circle class // by calling default constructor Circle circleObject = new Circle(); System.out.println(circleObject.radius); } }Output
0
Here, we haven't defined any constructor for class Circle. Hence, the Java compiler automatically creates the default constructor with no argument. The default constructor initializes any uninitialized member variables with following default values based on it's data type.
Data Type | Default Value |
---|---|
boolean | false |
byte | 0 |
short | 0 |
int | 0 |
long | 0L |
char | \u0000 |
float | 0.0f |
float | 0.0f |
double | 0.0d |
object | null |
String | null |
No Argument Constructor
A constructor without any argument is known as no argument constructor. However it can have code inside constructor's body unlike default constructor. It is not automatically
created by compiler, it is written by programmer within class body. We create no argument constructor to initialize the member varibles of all objects with a constant value.
Here is an example program to create an object of a class using no-arg constructor for object initialization.
class Box { int length; int width; int height; Box() { // Initialize member variables // of every Box object length = 10; width = 10; height = 5; System.out.println("Inside Constructor"); } public void printDimension() { System.out.println(length + "," + width + "," + height); } } public class NoArgumentConstructor { public static void main(String[] args) { // Creating objects of Box class // by calling no arg constructor Box box1 = new Box(); Box box2 = new Box(); box1.printDimension(); box2.printDimension(); } }Output
Inside Constructor Inside Constructor 10,10,5 10,10,5
Here, we have defined a no-arg constructor for class Box. When we create objects of Box class by calling no-arg constructor, it initializes memebr variable's of each object.
Parameterized constructor
A constructor method having arguments is knows as parameterized constructor. A parameterized constructor can take any number of parameters. We use parameterized constructors to initialize the member variables of an object during object creation.
A parameterized constructor is used to initialize different objects with different values during object creation. However, you can provide the same initial values for each object also.
class Board { int length; int width; Board(int len, int wid) { length = len; width = wid; System.out.println("Inside Constructor"); } public void printDimension() { System.out.println(length + "," + width); } } public class ParameterizedConstructor { public static void main(String[] args) { // Creating objects of Box class // by calling no arg constructor Board board1 = new Board(10, 20); Board board2 = new Board(4, 5); board1.printDimension(); board2.printDimension(); } }Output
Inside Constructor Inside Constructor 10,20 4,5
Copy Constructor
A constructor that accepts an object of its own type as a parameter and copies the data members is called a copy constructor. Copy constructors are used when we want to create a create a copy of an existing object.
For Example:class Board { int length; int width; Board (Board b) { length = b.length; width = b.width; } }
Constructor Overloading in Java
Like any other member method, we can overload constructor methods also. We can create multiple constructors having either different numbers of arguments or arguments of different data types. We use different constructors when we want to initialize some or all of member variables of an object. This is called constructors overloading.
class Board { int length; int width; Board() { System.out.println("No Arg Constructor"); } Board(int len) { length = len; System.out.println("One Arg Constructor"); } Board(int len, int wid) { length = len; width = wid; System.out.println("Two Arg Constructor"); } public void printDimension() { System.out.println(length + "," + width); } } public class ParameterizedConstructor { public static void main(String[] args) { Board board1 = new Board(); board1.printDimension(); Board board2 = new Board(5); board2.printDimension(); Board board3 = new Board(10, 20); board3.printDimension(); } }Output
No Arg Constructor 0,0 One Arg Constructor 5,0 Two Arg Constructor 10,20
In above example, we have defined three constructors of class Board with different number of arguments. You can call any one constructor by passing appropriate arguments to create objects of Board class.
Best Practices of Using Constructors
- Initialize All Attributes : Ensure that all attributes of the class are initialized within the constructor. This helps avoid unexpected behavior due to uninitialized variables.
- Keep Constructors Simple : Constructors should focus on initializing the object's state. Complex operations or business logic should be placed in separate methods.
- Avoid Code Duplication : If multiple constructors perform similar tasks, consider having one constructor call another to avoid code duplication.
- Use this Keyword : Use the this keyword to distinguish between attributes and parameters with the same name.
Conclusion
Constructors are a fundamental part of Java programming, ensuring that objects are properly initialized when created. Understanding the types of constructors, their rules, and best practices is essential for writing clean, efficient, and maintainable code. As you continue to explore Java development, leverage constructors to create objects with meaningful initial states and consider the design patterns and practices discussed in this tutorial to enhance the quality of your code.