Array in C++ is a collection of fixed size data belongings to the same data type. An array is a data structure provided in C++ which can store a number of variables of same data type in sequence. These similar elements could be of type int, float, double, char etc. All array elements are stored in the contiguous memory locations.
For Example :int score[100]; /* Integer array of size 100 */ char name[10]; /* character array of size 10 */
C++ programming language provides support for array data structure, which solves the problem of storing N similar data. We can declare an integer array of length 100 to store marks of 100 students.
int studentMarks[100];
In the above array declaration, we declared an array of name "studentMarks" which can store maximum of 100 integer data in contiguous memory locations. We can access the individual elements of an array using their index.
- studentMarks[0] refers to the first element of array.
- studentMarks[1] refers to the second element of array.
- studentMarks[99] refers to the last element of array which is 100th element.
Suppose, we have an integer array of size 7 whose name is score. The base address or starting address of array score is 1000(base address is same as address of first element score[0]) and the size of int be 2 bytes. Then, the address of second element(score[1]) will be 1002, address of third element(score[2]) will be 1004 and so on.
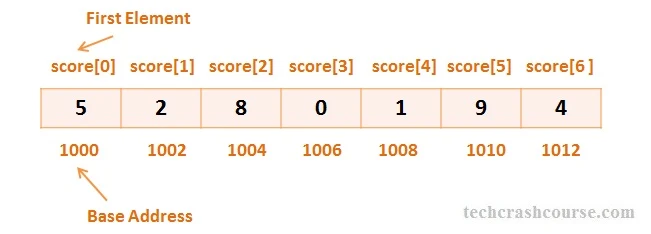
- An array is a collection of variables of same data types.
- The size of array must be a constant integral value.
- Array is a random access data structure. you can access any element of array in just one statement.
- All elements of array are stored in the contiguous memory locations.
- * Individual elements in an array can be accessed by the name of the array and an integer enclosed in square bracket called subscript/index variable like employeeSalary[5].
- The first element in an array is at index 0, whereas the last element is at index (size_of_array - 1)
- Random Access : We can access any elements of array in O(1) time complexity.
- Less amount of code : Using array we can aggregate N variables of same data type in a single data structure. Otherwise we have to declare N individual variables.
- Easy access of elements : We can access any element of array using array name and index. We can access all elements serially by iterating from index 0 to size-1 using a loop.
- Easy to implement algorithms : Certain algorithms can be easily implemented using array like searching and sorting, finding maximum and minimum elements.
How to declare an array in C++
Like any other variable in C++, we must declare an array before using it. Below is the syntax of declaring an 1D array.
- array_name : It is a valid C++ identifier.
- array_size : It is the maximum number of elements which can be stored in array. It must be an integer constant greater than zero.
- data_type : It represents the data type of each elements to be stored in array.
An Array of 10 Integers int age[10]; An Array of 50 Characters. char address[50]; Two Dimensional Array of Integers. int maze[20][20];
How to initialize an array in C++ programming
Arrays in C++ programming language is not initialized automatically at the time of declaration. By default, array elements contain garbage value. Like any other variable in C++, we can initialize arrays at the time of declaration.
Array Initialization by Specifying Array Sizeint marks[7] = {5,2,9,1,1,4,7};
Array Initialization Without Specifying Array Size
int marks[] = {5,2,9,1,1,4,7};
In above declaration, compiler counts the number Of elements inside curly braces{} and determines the size of array score. Then, compiler will create an array of size 7 and initialize it with value provided between curly braces{} as discussed above.
Common Array Operations
- Updating Array Elements : Array elements can be updated by assigning new values to them.
int numbers[3] = {1, 2, 3}; // Updating array elements numbers[1] = 10; // Result: {1, 10, 3}
- Iterating Through an Array : Loops are commonly used to iterate through arrays.
int numbers[5] = {1, 2, 3, 4, 5}; // Iterating through an array for (int i = 0; i < 5; ++i) { std::cout << numbers[i] << " "; } // Output: 1 2 3 4 5
- Finding the Size of an Array : While arrays don't inherently carry information about their size, the sizeof operator can be used to find the total size in bytes.
int numbers[5] = {1, 2, 3, 4, 5}; // Finding the size of an array int size = sizeof(numbers) / sizeof(numbers[0]); // Result: 5
- Multidimensional Arrays : C++ supports multidimensional arrays, providing a way to represent matrices and higher-dimensional structures.
// Declaration of a 2D array int matrix[3][3] = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} };
Advanced Array Topics in C++
- Array of Objects : Arrays can store objects of user-defined types.
// Array of objects class Point { public: int x, y; }; Point points[3] = {{1, 2}, {3, 4}, {5, 6}};
- Pointers and Arrays : Arrays and pointers are closely related in C++. The name of an array can be treated as a pointer to its first element.
int numbers[5] = {1, 2, 3, 4, 5}; // Using pointers with arrays int* ptr = numbers; // Equivalent to int* ptr = &numbers[0]
- Dynamic Arrays (std::vector) : For dynamic sizing, the C++ Standard Library provides std::vector, a dynamic array implementation.
#include <vector> // Dynamic array using std::vector std::vector<int> dynamicArray = {1, 2, 3, 4, 5};
std::vector dynamically adjusts its size and provides convenient functions for array manipulation. - Standard Library Algorithms : The
header in the C++ Standard Library offers powerful algorithms that can be applied to arrays. #include <algorithm> int numbers[5] = {4, 2, 5, 1, 3}; // Sorting an array using std::sort std::sort(numbers, numbers + 5); // Result: {1, 2, 3, 4, 5}
Conclusion
Arrays form the backbone of data storage and manipulation in C++. By understanding their syntax, operations, and advanced features, you gain a powerful tool for organizing and processing data efficiently. Whether dealing with static arrays or embracing the dynamic capabilities of std::vector, arrays empower you to craft robust and expressive code.