The decision making statements in C language are used to perform operations on the basis of condition and when program encounters the situation to choose a particular statement among many statements.
Decision making statements lets you evaluate one or more conditions and then take decision whether to execute a set of statements or not.
By using decision making statements you can execute statements or select some statements to be executed base on outcome of condition(whether it is true or false). The outcome of conditional statement must be either true(In C, all non-zero and non-null values are considered as true) or false((In C, zero and null value is considered as false).
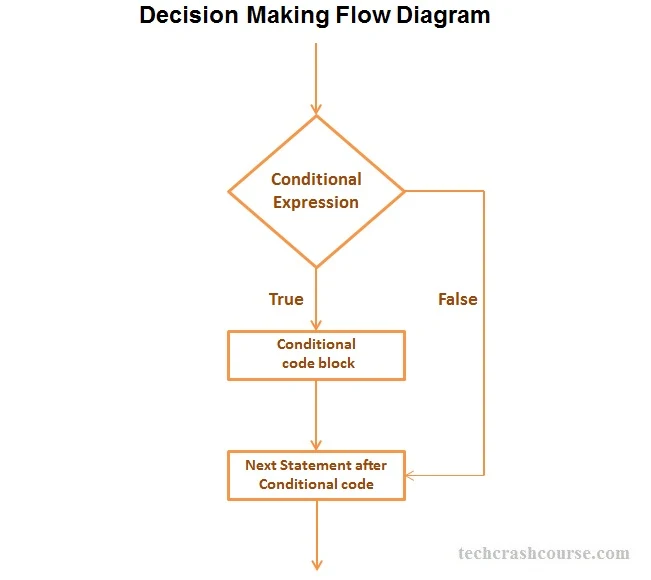
If Statement in C
The if statement in C programming language is used to execute a block of code only if condition is true.
Syntax of If Statement
/* code to be execute if the condition_expression is true */
statements;
}
The if statement checks whether condition_expression is true or false. If the condition_expression is true, then the block of code inside the if statement is executed but if condition_expression is false, then the block of code inside the if statement is ignored and the next statement after if block is executed.
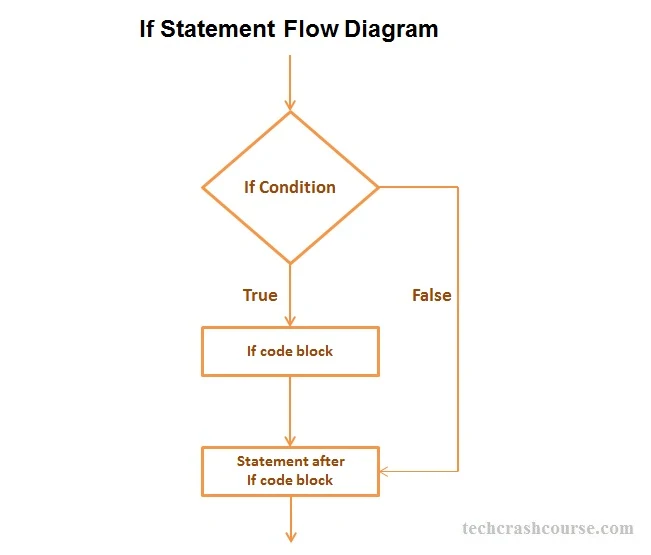
C Program to print Pass or Failed using If Condition Statement
#include <stdio.h> int main(){ int marks; printf("Enter your Marks : "); scanf("%d", &marks); /* Using if statement to decide whether a student failed in examination or not*/ if(marks < 40){ printf("You Failed :(\n"); } if(marks >= 40){ printf("Congrats You Passed :)\n"); } return(0); }Above program check whether a student passed or failed in examination using if statement.
Output
Enter your Marks : 70 Congrats You Passed :)
Enter your Marks : 35 You Failed :(
If Else Statement in C
The if else statement in C programming language is used to execute a set of statements if condition is true and execute another set of statements when condition is false. Only either if block or else block of code gets executed(not both) depending on the outcome of condition.
Syntax of if else statement
/* code to be execute if the condition_expression is true */
statements;
} else {
/* code to be execute if the condition_expression is false */
statements;
}
The if else statement checks whether condition_expression is true or false. If the condition_expression is true, then the block of code inside the if statement is executed but if condition_expression is false, then the block of code inside the else statement is executed.
Both if and else block of statements can never execute at a time.
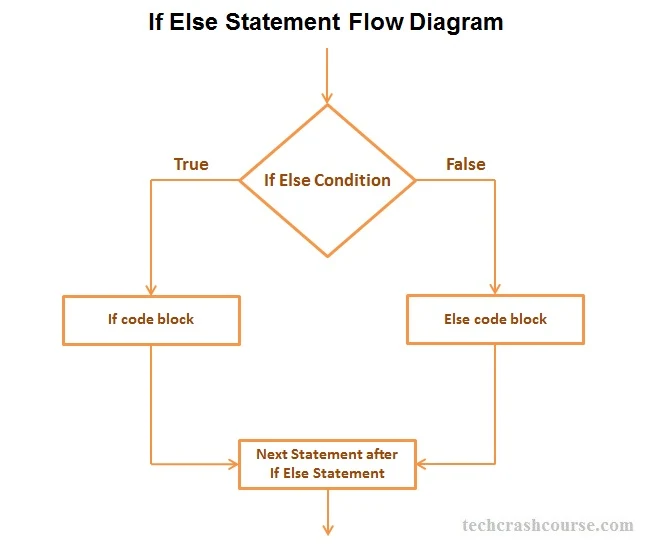
C Program to show use of If Else Control Statement
#include<stdio.h> int main() { int marks; printf("Enter your Marks : "); scanf("%d", &marks); /* Using if else statement to decide whether a student passed or failed in examination */ if(marks < 40){ printf("You Failed :(\n"); } else { printf("Congrats You Passed :)\n"); } return(0); }Above program check whether a student passed or failed in examination using if else statement.
Output
Enter your Marks : 45 Congrats You Passed :)
Enter your Marks : 25 You Failed :(
- The condition_expression is a boolean expression. It must evaluate to true or false value(In C, all non-zero and non-null values are considered as true and zero and null value is considered as false).
- We may use more than one condition inside if else statement.
For Example:
if(condition_one && condition_two) {
/* if code block */
} else {
/* else code block */
}
- Opening and Closing Braces are optional, If the block of code of if else statement contains only one statement.
For Example:
if(condition_one && condition_two)
statement1;
else
statement2
In above example only statement1 will gets executed if condition_expression is true otherwise statement2.
Best Practices for Using If-Else Statements in C
- Use Descriptive Variable and Function Names : Choose meaningful names for variables and functions to enhance code readability. Descriptive names make it easier to understand the purpose of conditions.
// Better naming int isPositive(int number) { return (number > 0); } // Usage if (isPositive(x)) { // Code for positive numbers } else { // Code for non-positive numbers }
- Avoid Nesting Too Deeply : Excessive nesting can make code harder to read and understand. Consider refactoring complex conditions or using helper functions to improve code clarity.
// Excessive nesting if (condition1) { if (condition2) { if (condition3) { // Code } } } // Improved structure if (condition1 && condition2 && condition3) { // Code }
- Be Mindful of Code Duplication : When similar code blocks are repeated in multiple branches of an if-else statement, consider refactoring to avoid code duplication.
// Code duplication if (condition1) { // Code A } else { // Code A } // Refactored if (condition1 || condition2) { // Code A } if (!condition1 && condition2) { // Code B }
- Use Parentheses for Complex Conditions : When combining multiple conditions, use parentheses to clarify the intended logic and avoid ambiguity.
// Ambiguous condition if (x > 0 || y > 0 && z > 0) { // Code } // Clarified condition if ((x > 0 || y > 0) && z > 0) { // Code }
- Test Edge Cases : Test your if-else statements with boundary values and edge cases to ensure they handle all possible scenarios correctly.
// Testing edge cases int x = 0; if (x > 0) { // Positive case } else if (x < 0) { // Negative case } else { // Zero case }
- Document Complex Conditions : If your conditions involve complex logic, consider adding comments to explain the rationale behind the conditions.
// Complex condition with documentation if (isWeekday(day) && (temperature > 0 || precipitation < 0.1)) { // Code for favorable weather conditions }
Conclusion
In conclusion, mastering the if-else statement is a crucial skill for any C programmer. This powerful construct allows you to create dynamic and responsive programs by making decisions based on different conditions. Understanding how to structure and nest if-else statements provides you with the flexibility to handle complex decision-making processes in your code. Additionally, the use of logical operators and relational expressions enhances the expressiveness of your conditions, allowing you to create more sophisticated and accurate decision structures.
Remember that the if-else statement is just one part of the broader control flow mechanisms in C. Combining it with loops, switch statements, and functions allows you to create robust and efficient programs that can handle a variety of scenarios.